According to StackOverflow, Python is the fastest growing programming language. For example, one of the
Forbes reports says that Python usage grew by 456%. Python is used by Netflix, IBM, and thousands of companies around the world. Let's not forget about Dropbox. The services of this company are also written in Python. According to
Dice research, knowledge in the field of Python is very much in demand today, and
the popularity
index of programming languages suggests that Python is today the most popular language in the world. If you compare Python with other languages, it turns out that it has the following strengths:
- Compatible with the vast majority of platforms and operating systems.
- The presence of many open source frameworks and tools.
- Code that is easy to read and maintain.
- Reliable standard library.
- Standard development mechanism through testing.
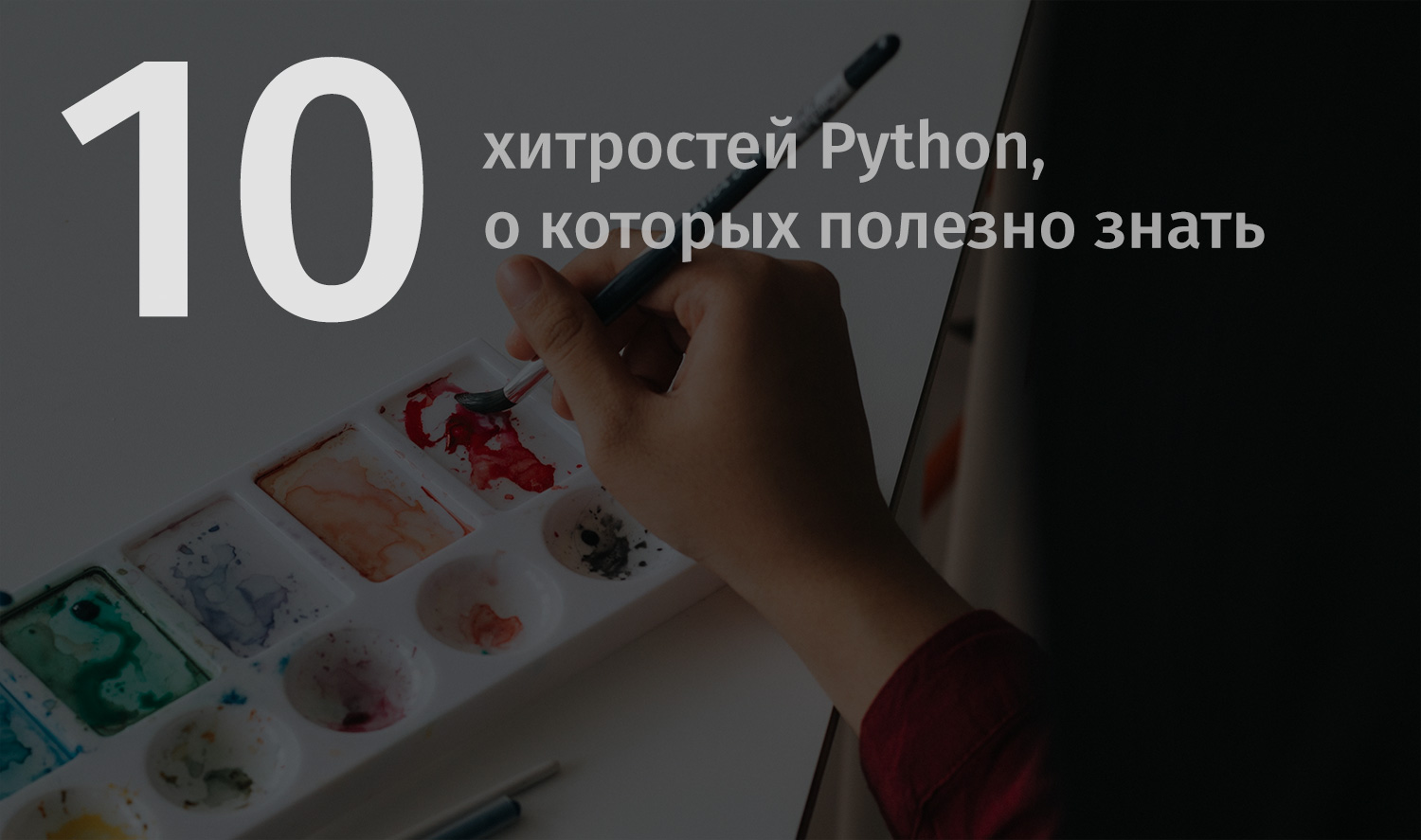
Python Tricks
Here I would like to bring to your attention 10 useful tips regarding Python programming. They are quite capable of helping you in your daily work.
▍1. String concatenation
If you need to concatenate a list of strings, you can do this in a
for
loop, adding one line at a time to the final result. However, this approach will be very inefficient, especially if the list is long enough. In Python, strings are immutable entities. As a result, each string concatenation operation means you need to copy a couple of lines to a new line.
A more efficient approach to solving this problem is to use the
join()
function:
characters = ['p', 'y', 't', 'h', 'o', 'n'] word = "".join(characters) print(word)
▍2. Using List Generators
List generators are used to create new lists from other iterable objects. Since the generator returns lists, its description is an expression, enclosed in square brackets, executed for each element of the list. This also includes a description of the
for
loop that goes through each element. List generators allow you to speed up work with lists due to the fact that the Python interpreter is optimized for templates that are repeated when the list is traversed.
As an example, consider finding the squares of the first five integers using a list generator:
m = [x ** 2 for x in range(5)] print(m)
Now we find the numbers that appear in each of the two lists:
list_a = [1, 2, 3, 4] list_b = [2, 3, 4, 5] common_num = [a for a in list_a for b in list_b if a == b] print(common_num)
▍3. Iterate over lists with enumerate ()
The
enumerate()
method adds numbering to the iterated collection and returns an object that generates pairs of elements consisting of the index of the element and this element itself.
Let's solve the classic FizzBuzz problem offered at interviews. Here is the condition for this task: “Write a program that displays a list of numbers. In this case, instead of numbers that are multiples of 3, fizz is displayed, instead of numbers that are multiples of 5 - buzz, and instead of numbers that are multiples of 3 and 5 - fizzbuzz. "
numbers = [30, 42, 28, 50, 15] for i, num in enumerate(numbers): if num % 3 == 0 and num % 5 == 0: numbers[i] = 'fizzbuzz' elif num % 3 == 0: numbers[i] = 'fizz' elif num % 5 == 0: numbers[i] = 'buzz' print(numbers)
▍4. Using zip () with lists
Suppose you need to combine several lists of the same length and display the resulting list. As in other cases, a similar problem can be solved, so to speak, “on the forehead”, or you can use something like the universal
zip()
function:
countries = ['France', 'Germany', 'Canada'] capitals = ['Paris', 'Berlin', 'Ottawa'] for country, capital in zip(countries,capitals): print(country, capital)
▍5. Using the itertools module
The
itertools
Python module is a set of tools for working with iterators. This module includes many tools for generating various sequences. Here, as an example, consider the
itertools.combinations()
method. This method is used to create combinations. There are also tools for grouping input values.
Let's consider a real example in order to understand the above.
Imagine that in a certain tournament four teams participate. In the group round, matches of each team against each of the other teams are held. Your task is to generate all possible combinations of teams that will play against each other.
Take a look at the following code:
import itertools friends = ['Team 1', 'Team 2', 'Team 3', 'Team 4'] list(itertools.combinations(friends, r=2))
Here you need to pay attention to the fact that the order of values is unimportant. Since the combinations
('Team 1', 'Team 2')
and
('Team 2', 'Team 1')
represent the same pair of teams, only one of them will be included in the final list. Similarly, you can use the
itertools.permutations()
method and other methods of this module. A detailed guide to
itertools
can be found
here .
▍6. Using Python Collections
Python collections are container data types. In particular, these are lists, sets, tuples, dictionaries. The
collections
module provides the developer with high-performance data types that help improve the code, make it cleaner, and make it easier to work with. This module contains many useful methods. Here we look at the
Counter()
method.
This method takes an iterable object, such as a list or tuple, and returns a dictionary containing information about the number of different objects in the list being examined (Counter Dictionary). The keys to such a dictionary are unique elements represented in the iterable object, and the values are the quantities of such elements.
To create a
Counter
object, you need to pass an iterable object (list, for example) to the
Counter()
method:
import collections count = collections.Counter(['a','b','c','d','b','c','d','b']) print(count)
Details on the
collections
module can be found
here .
▍ 7. Convert two lists to a dictionary
Suppose we have two lists. One of them contains the names of students, and the second contains their grades. How to convert these two lists to a dictionary? If we resort to the
zip()
function for this, then this problem can be solved as follows:
students = ["Peter", "Julia", "Alex"] marks = [84, 65, 77] dictionary = dict(zip(students, marks)) print(dictionary)
▍8. Using Generator Functions
Generator functions are functions that behave like iterators. They allow the programmer to quickly and easily create neat iterators. Consider an example that reveals this idea.
Suppose we need to find the sum of the squares of the first 100,000,000 integers starting at 1.
This task seems to be simple. It can be solved without problems using list generators. However, the problem here is the large amount of input data. Take a look at the following code:
import time t1 = time.clock() sum([i * i for i in range(1, 100000000)]) t2 = time.clock() time_diff = t2 - t1 print(f"It took {time_diff} Secs to execute this method")
If we need to find the sum of the squares for an even larger number of numbers, then it turns out that this technique does not work well for finding them, because it takes a lot of time to find a solution. In this situation, Python generator functions will come to our aid. In our case, in order to switch from using list generators to generator functions, it is enough to change the square brackets in the generator expression to round ones. We do this and find the time needed to solve the problem:
import time t1 = time.clock() sum((i * i for i in range(1, 100000000))) t2 = time.clock() time_diff = t2 - t1 print(f"It took {time_diff} Secs to execute this method")
As you can see, now it takes less time to solve the same problem. And with increasing input data, this effect will be even more noticeable.
Here is material about generator functions.
▍ 9. Returning multiple values from a function
Python has the ability to return multiple values from a function. This is not found in many other popular programming languages. To return multiple values from a function, they must be separated by commas. Based on this list of values, Python will create a tuple and return it to where the function was called. Here is an example:
def multiplication_division(num1, num2): return num1*num2, num1/num2 product, division = multiplication_division(15, 3) print("Product =", product, "Quotient =", division)
▍ 10. Using the sorted () Function
In Python, it is very easy to sort certain data sequences using the built-in
sorted()
function, which takes care of solving all the related tasks. This function sorts any sequences (lists, tuples) and always returns a list with sorted elements. Consider an example of sorting a list of numbers in ascending order:
sorted([3,5,2,1,4])
And here is an example of sorting a list of rows in descending order:
sorted(['france', 'germany', 'canada', 'india', 'china'], reverse=True)
Summary
This article has presented 10 useful Python programming tips that you might find useful in your daily work. We hope you find among them something that will benefit you.
Dear readers! Experienced Python developers are encouraged to talk about the useful Python features that they regularly use.
