Intel Edison . , - , Intel Edison Physical computing (Physical computing — . , ). Intel Edison
Cylon.js, JavaScript- , (IoT).
. , Cylon.js , Intel IoT Developer Kit. , « », , .. . , Intel Edison, .
Intel Edison, , :
Cylon.js , — . , , LCD , API. IoT-, .
, , Intel Edison. , 7-15 1 .
1.
. , . , - «Hello, World» IoT, . - . Grove . , «+» (), «+» Grove.
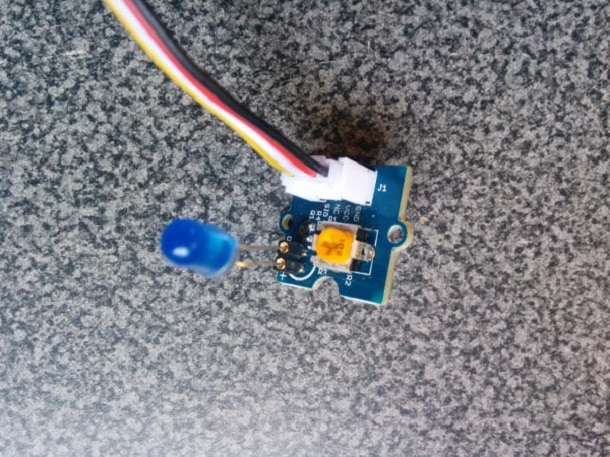
main.js "use strict";
var cylon = require("cylon");
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
led: { driver: "led", pin: 3, connection: "edison" }
},
work: function() {
var that = this;
setInterval(function() {
that.led.toggle()
}, 1000);
}
}).start();
. «main.js» Intel® XDK IoT Edition, .
.
2.
. , . Cylon.js . - jQuery, , «MouseOver». Cylon.js , . , «push» «release» («» «»). , , .
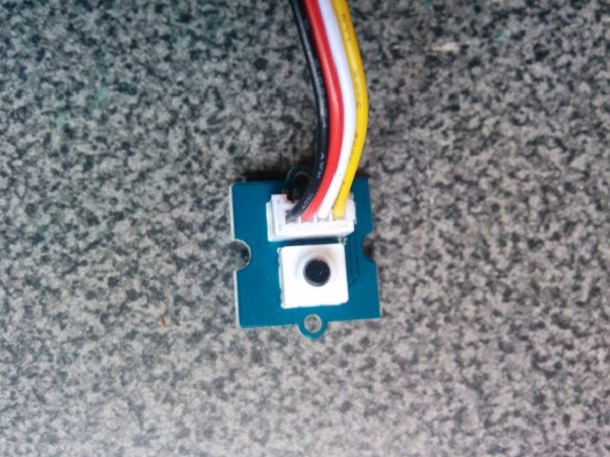
main.js"use strict";
var cylon = require("cylon");
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
},
setup: function() {
this.led.turnOff();
},
work: function() {
var that = this;
that.setup();
that.button.on('push', function() {
that.led.turnOn();
});
that.button.on('release', function() {
that.led.turnOff();
});
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
, .
3.
-. , , , . - RGB- . .
- , , I2C. I2C- . «i2c» Grove-.
-. . button.on(“release”) (“push”) .
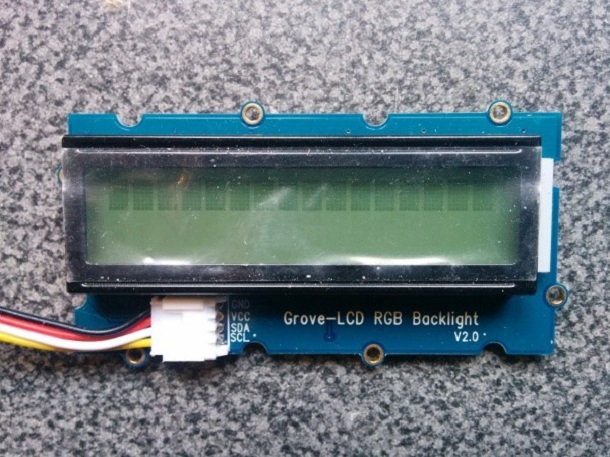
main.js"use strict";
var cylon = require("cylon");
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
screen: { driver: "upm-jhd1313m1", connection: "edison" }
},
writeMessage: function(message, color) {
var that = this;
var str = message.toString();
while (str.length < 16) {
str = str + " ";
}
console.log(message);
that.screen.setCursor(0,0);
that.screen.write(str);
switch(color)
{
case "red":
that.screen.setColor(255, 0, 0);
break;
case "green":
that.screen.setColor(0, 255, 0);
break;
case "blue":
that.screen.setColor(0, 0, 255);
break;
default:
that.screen.setColor(255, 255, 255);
break;
}
},
reset: function() {
this.writeMessage("Doorbot ready");
this.led.turnOff();
},
work: function() {
var that = this;
that.reset();
that.button.on('push', function() {
that.led.turnOn();
that.writeMessage("Lights On", "blue");
});
that.button.on('release', function() {
that.reset();
});
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
, -.
4 — Web API
Cylon.js HTTP API, - REST. Cylon.js API . . «http», «socketio», «mqtt». .
HTTP API Robeaux (
http://robeaux.io), , -, React. .
API- Cylon.js . Common Protocol for Programming Physical Input/Output «cppp.io».
, «Doorbot», «/api/robots/doorbot/devices/led». «/api/robots/<robot name>/devices/<device name>» Cylon.js API .
main.js"use strict";
var cylon = require("cylon");
cylon.api({
host: "0.0.0.0",
port: "3000",
ssl: false
});
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
screen: { driver: "upm-jhd1313m1", connection: "edison" }
},
writeMessage: function(message, color) {
var that = this;
var str = message.toString();
while (str.length < 16) {
str = str + " ";
}
console.log(message);
that.screen.setCursor(0,0);
that.screen.write(str);
switch(color)
{
case "red":
that.screen.setColor(255, 0, 0);
break;
case "green":
that.screen.setColor(0, 255, 0);
break;
case "blue":
that.screen.setColor(0, 0, 255);
break;
default:
that.screen.setColor(255, 255, 255);
break;
}
},
reset: function() {
this.writeMessage("Doorbot ready");
this.led.turnOff();
},
work: function() {
var that = this;
that.reset();
that.button.on('push', function() {
that.led.turnOn();
that.writeMessage("Lights On", "blue");
});
that.button.on('release', function() {
that.reset();
});
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
IP- , 3000 ( Robeaux). :
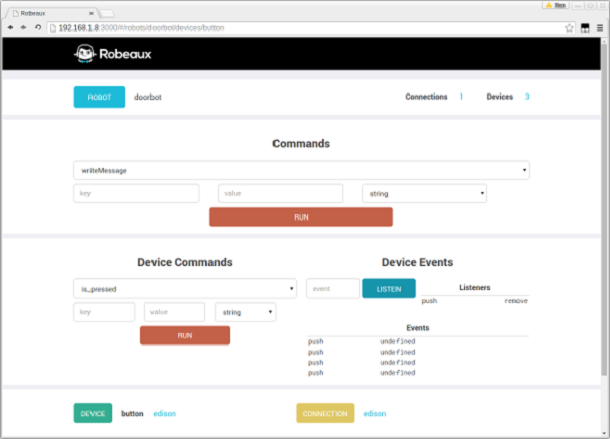
«doorbot». . «button». .
«Device Events» «push», «Listen». . , -. , .
5.
. . Cylon.js . , .
touch.on(“push”). .
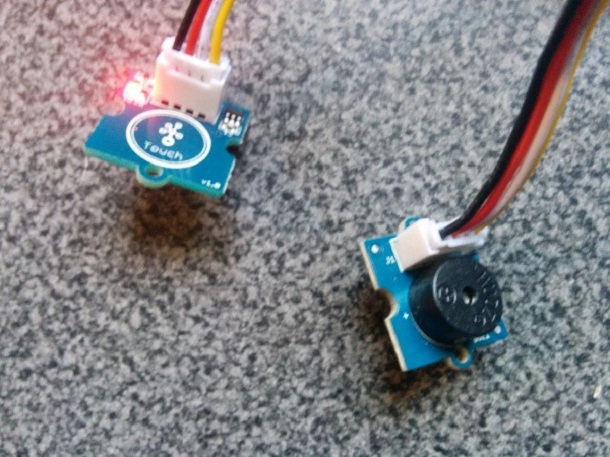
.
main.js"use strict";
var cylon = require("cylon");
cylon.api({
host: "0.0.0.0",
port: "3000",
ssl: false
});
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
buzzer: { driver: "direct-pin", pin: 7, connection: "edison" },
touch: { driver: "button", pin: 8, connection: "edison" },
screen: { driver: "upm-jhd1313m1", connection: "edison" }
},
doorbell: function() {
var that = this;
that.buzzer.digitalWrite(1);
that.writeMessage("Doorbell pressed", "green");
setTimeout(function() {
that.reset();
}, 1000);
},
writeMessage: function(message, color) {
var that = this;
var str = message.toString();
while (str.length < 16) {
str = str + " ";
}
console.log(message);
that.screen.setCursor(0,0);
that.screen.write(str);
switch(color)
{
case "red":
that.screen.setColor(255, 0, 0);
break;
case "green":
that.screen.setColor(0, 255, 0);
break;
case "blue":
that.screen.setColor(0, 0, 255);
break;
default:
that.screen.setColor(255, 255, 255);
break;
}
},
reset: function() {
this.writeMessage("Doorbot ready");
this.led.turnOff();
this.buzzer.digitalWrite(0);
},
work: function() {
var that = this;
that.reset();
that.button.on('push', function() {
that.led.turnOn();
that.writeMessage("Lights On", "blue");
});
that.button.on('release', function() {
that.reset();
});
that.touch.on('push', function() {
that.doorbell();
});
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
, , .
6.
() . , . . .
. , , , . , .
, , 180 . , . , . .
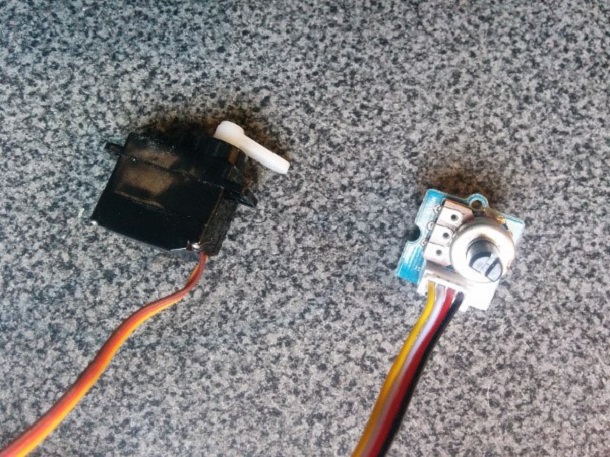
:
main.js"use strict";
var cylon = require("cylon");
cylon.api({
host: "0.0.0.0",
port: "3000",
ssl: false
});
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
servo: { driver: "servo", pin: 5, connection: "edison" },
buzzer: { driver: "direct-pin", pin: 7, connection: "edison" },
touch: { driver: "button", pin: 8, connection: "edison" },
dial: { driver: "analogSensor", pin: 0, connection: "edison" },
screen: { driver: "upm-jhd1313m1", connection: "edison" }
},
turnLock: function(val) {
var that = this;
var currentAngle = that.servo.currentAngle();
var angle = val.fromScale(0, 1023).toScale(0,180) | 0;
if (angle <= currentAngle - 3 || angle >= currentAngle + 3) {
console.log("turning lock:", angle);
that.servo.angle(angle);
}
},
doorbell: function() {
var that = this;
that.buzzer.digitalWrite(1);
that.writeMessage("Doorbell pressed", "green");
setTimeout(function() {
that.reset();
}, 1000);
},
writeMessage: function(message, color) {
var that = this;
var str = message.toString();
while (str.length < 16) {
str = str + " ";
}
console.log(message);
that.screen.setCursor(0,0);
that.screen.write(str);
switch(color)
{
case "red":
that.screen.setColor(255, 0, 0);
break;
case "green":
that.screen.setColor(0, 255, 0);
break;
case "blue":
that.screen.setColor(0, 0, 255);
break;
default:
that.screen.setColor(255, 255, 255);
break;
}
},
reset: function() {
this.writeMessage("Doorbot ready");
this.led.turnOff();
this.buzzer.digitalWrite(0);
},
work: function() {
var that = this;
that.reset();
that.button.on('push', function() {
that.led.turnOn();
that.writeMessage("Lights On", "blue");
});
that.button.on('release', function() {
that.reset();
});
that.dial.on('analogRead', function(val) {
that.turnLock(val);
});
that.touch.on('push', function() {
that.doorbell();
});
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
, .
7.
. . , , . , .
UMP, Cylon.js. UPM Intel, . «Grove — Temperature Sensor».
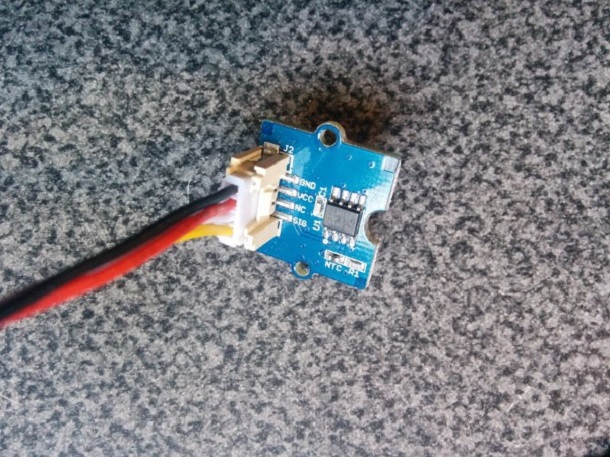
.
main.js"use strict";
var cylon = require("cylon");
cylon.api({
host: "0.0.0.0",
port: "3000",
ssl: false
});
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
servo: { driver: "servo", pin: 5, connection: "edison" },
buzzer: { driver: "direct-pin", pin: 7, connection: "edison" },
touch: { driver: "button", pin: 8, connection: "edison" },
dial: { driver: "analogSensor", pin: 0, connection: "edison" },
temp: { driver: "upm-grovetemp", pin: 1, connection: "edison" },
screen: { driver: "upm-jhd1313m1", connection: "edison" }
},
fireAlarm: function() {
var that = this;
var deg = that.temp.value();
console.log("current temp:", deg);
if (deg >= 30) {
that.writeMessage("Fire alarm!", "red");
that.buzzer.digitalWrite(1);
setTimeout(function() {
that.buzzer.digitalWrite(0);
}, 200);
}
},
turnLock: function(val) {
var that = this;
var currentAngle = that.servo.currentAngle();
var angle = val.fromScale(0, 1023).toScale(0,180) | 0;
if (angle <= currentAngle - 3 || angle >= currentAngle + 3) {
console.log("turning lock:", angle);
that.servo.angle(angle);
}
},
doorbell: function() {
var that = this;
that.buzzer.digitalWrite(1);
that.writeMessage("Doorbell pressed", "green");
setTimeout(function() {
that.reset();
}, 1000);
},
writeMessage: function(message, color) {
var that = this;
var str = message.toString();
while (str.length < 16) {
str = str + " ";
}
console.log(message);
that.screen.setCursor(0,0);
that.screen.write(str);
switch(color)
{
case "red":
that.screen.setColor(255, 0, 0);
break;
case "green":
that.screen.setColor(0, 255, 0);
break;
case "blue":
that.screen.setColor(0, 0, 255);
break;
default:
that.screen.setColor(255, 255, 255);
break;
}
},
reset: function() {
this.writeMessage("Doorbot ready");
this.led.turnOff();
this.buzzer.digitalWrite(0);
},
work: function() {
var that = this;
that.reset();
that.button.on('push', function() {
that.led.turnOn();
that.writeMessage("Lights On", "blue");
});
that.button.on('release', function() {
that.reset();
});
that.dial.on('analogRead', function(val) {
that.turnLock(val);
});
that.touch.on('push', function() {
that.doorbell();
});
setInterval(function() {
that.fireAlarm();
}, 1000);
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
.
8.
. - - , , , . , .
. , 0 1023. , , .
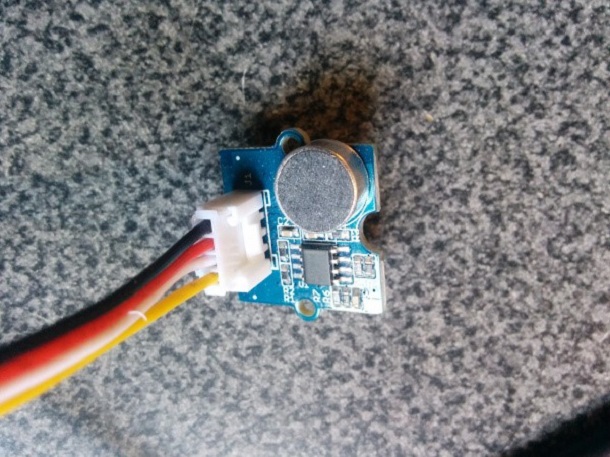
.
main.js"use strict";
var cylon = require("cylon");
cylon.api({
host: "0.0.0.0",
port: "3000",
ssl: false
});
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
servo: { driver: "servo", pin: 5, connection: "edison" },
buzzer: { driver: "direct-pin", pin: 7, connection: "edison" },
touch: { driver: "button", pin: 8, connection: "edison" },
dial: { driver: "analogSensor", pin: 0, connection: "edison" },
temp: { driver: "upm-grovetemp", pin: 1, connection: "edison" },
sound: { driver: "analogSensor", pin: 2, connection: "edison" },
screen: { driver: "upm-jhd1313m1", connection: "edison" }
},
fireAlarm: function() {
var that = this;
var deg = that.temp.value();
console.log("current temp:", deg);
if (deg >= 30) {
that.writeMessage("Fire alarm!", "red");
that.buzzer.digitalWrite(1);
setTimeout(function() {
that.buzzer.digitalWrite(0);
}, 200);
}
},
detectSound: function(val) {
var that = this;
if (val >= 450) {
console.log("Sound detected:", val)
that.writeMessage("Sound detected", "blue");
that.led.turnOn();
setTimeout(function() {
that.reset();
}, 500);
}
},
turnLock: function(val) {
var that = this;
var currentAngle = that.servo.currentAngle();
var angle = val.fromScale(0, 1023).toScale(0,180) | 0;
if (angle <= currentAngle - 3 || angle >= currentAngle + 3) {
console.log("turning lock:", angle);
that.servo.angle(angle);
}
},
doorbell: function() {
var that = this;
that.buzzer.digitalWrite(1);
that.writeMessage("Doorbell pressed", "green");
setTimeout(function() {
that.reset();
}, 1000);
},
writeMessage: function(message, color) {
var that = this;
var str = message.toString();
while (str.length < 16) {
str = str + " ";
}
console.log(message);
that.screen.setCursor(0,0);
that.screen.write(str);
switch(color)
{
case "red":
that.screen.setColor(255, 0, 0);
break;
case "green":
that.screen.setColor(0, 255, 0);
break;
case "blue":
that.screen.setColor(0, 0, 255);
break;
default:
that.screen.setColor(255, 255, 255);
break;
}
},
reset: function() {
this.writeMessage("Doorbot ready");
this.led.turnOff();
this.buzzer.digitalWrite(0);
},
work: function() {
var that = this;
that.reset();
that.button.on('push', function() {
that.led.turnOn();
that.writeMessage("Lights On", "blue");
});
that.button.on('release', function() {
that.reset();
});
that.dial.on('analogRead', function(val) {
that.turnLock(val);
});
that.sound.on('analogRead', function(val) {
that.detectSound(val);
});
that.touch.on('push', function() {
that.doorbell();
});
setInterval(function() {
that.fireAlarm();
}, 1000);
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
, , .
9. ()
. , - , . , , .
, .
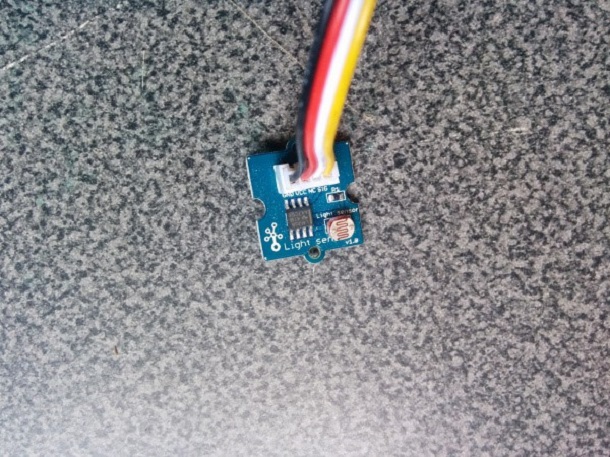
.
main.js"use strict";
var cylon = require("cylon");
cylon.api({
host: "0.0.0.0",
port: "3000",
ssl: false
});
cylon.robot({
name: "doorbot",
connections: {
edison: { adaptor: "intel-iot" }
},
devices: {
button: { driver: "button", pin: 2, connection: "edison" },
led: { driver: "led", pin: 3, connection: "edison" },
servo: { driver: "servo", pin: 5, connection: "edison" },
buzzer: { driver: "direct-pin", pin: 7, connection: "edison" },
touch: { driver: "button", pin: 8, connection: "edison" },
dial: { driver: "analogSensor", pin: 0, connection: "edison" },
temp: { driver: "upm-grovetemp", pin: 1, connection: "edison" },
sound: { driver: "analogSensor", pin: 2, connection: "edison" },
light: { driver: "analogSensor", pin: 3, connection: "edison" },
screen: { driver: "upm-jhd1313m1", connection: "edison" }
},
fireAlarm: function() {
var that = this;
var deg = that.temp.value();
console.log("current temp:", deg);
if (deg >= 30) {
that.writeMessage("Fire alarm!", "red");
that.buzzer.digitalWrite(1);
setTimeout(function() {
that.buzzer.digitalWrite(0);
}, 200);
}
},
detectSound: function(val) {
var that = this;
if (val >= 450) {
console.log("Sound detected:", val)
that.writeMessage("Sound detected", "blue");
that.led.turnOn();
setTimeout(function() {
that.reset();
}, 500);
}
},
detectLight: function(val) {
var that = this;
var date = new Date();
var currentHour = date.getHours();
if (currentHour > 19 && currentHour < 8 && val >= 450) {
console.log("Light detected:", val)
that.writeMessage("Light detected", "blue");
that.led.turnOn();
setTimeout(function() {
that.reset();
}, 500);
}
},
turnLock: function(val) {
var that = this;
var currentAngle = that.servo.currentAngle();
var angle = val.fromScale(0, 1023).toScale(0,180) | 0;
if (angle <= currentAngle - 3 || angle >= currentAngle + 3) {
console.log("turning lock:", angle);
that.servo.angle(angle);
}
},
doorbell: function() {
var that = this;
that.buzzer.digitalWrite(1);
that.writeMessage("Doorbell pressed", "green");
setTimeout(function() {
that.reset();
}, 1000);
},
writeMessage: function(message, color) {
var that = this;
var str = message.toString();
while (str.length < 16) {
str = str + " ";
}
console.log(message);
that.screen.setCursor(0,0);
that.screen.write(str);
switch(color)
{
case "red":
that.screen.setColor(255, 0, 0);
break;
case "green":
that.screen.setColor(0, 255, 0);
break;
case "blue":
that.screen.setColor(0, 0, 255);
break;
default:
that.screen.setColor(255, 255, 255);
break;
}
},
reset: function() {
this.writeMessage("Doorbot ready");
this.led.turnOff();
this.buzzer.digitalWrite(0);
},
work: function() {
var that = this;
that.reset();
that.button.on('push', function() {
that.led.turnOn();
that.writeMessage("Lights On", "blue");
});
that.button.on('release', function() {
that.reset();
});
that.dial.on('analogRead', function(val) {
that.turnLock(val);
});
that.sound.on('analogRead', function(val) {
that.detectSound(val);
});
that.light.on('analogRead', function(val) {
that.detectLight(val);
});
that.touch.on('push', function() {
that.doorbell();
});
setInterval(function() {
that.fireAlarm();
}, 1000);
}
}).start();
. «main.js» Intel® XDK IoT Edition, , .
, , .
, Cylon.js Intel Edison Intel® IoT Developer Kit, . , .
Intel Edison . . Cylon.js . ?
Intel IoT Developer Kit
IoT : Intel Edison + Intel XDK + JavaScript + Grove Kit