UPD
Link zum Github.
Kürzlich kam mir die Idee, ein einfaches Spiel mit einer minimalen Anzahl von Zeilen zu schreiben. Meine Wahl fiel auf Tetris. In diesem Artikel werde ich meinen Code beschreiben.
Zunächst ist anzumerken, dass ich in meine Implementierung nur die grundlegenden Funktionen aufgenommen habe:
- Bewegung der Figuren nach links / rechts;
- fallende Zahlen;
- Rotation der Figuren;
- gefüllte Zahlen löschen;
- das Ende des Spiels.
Fügen Sie dem Formular zunächst eine PictureBox hinzu und erstellen Sie einen Timer.
Auch für das Spiel benötigen Sie:
public const int width = 15, height = 25, k = 15;
Füllen Sie das Feld um die Ränder:
for (int i = 0; i < width; i++) field[i, height - 1] = 1; for (int i = 0; i < height; i++) { field[0, i] = 1; field[width - 1, i] = 1; }
Füllen Sie die Abbildung aus:
public void SetShape(){ Random x = new Random(DateTime.Now.Millisecond); switch (x.Next(7)){
Das Verfahren zum Zeichnen des „Glases“ in der PictureBox:
public void FillField(){ gr.Clear(Color.Black);
Ich verschiebe zuerst die Figuren und überprüfe dann, ob diese Option möglich ist oder nicht. Wenn an einer Stelle ein Fehler auftritt (die Figur befindet sich außerhalb des Feldes oder überlagert die bereits im Feld befindliche Figur), kehrt die Figur an ihre ursprüngliche Stelle zurück. Zu diesem Zweck habe ich eine Funktion geschrieben, die true zurückgibt, wenn ein Fehler im Feld gefunden wurde, oder false, wenn kein Fehler vorliegt:
public bool FindMistake(){ for (int i = 0; i < 4; i++) if (shape[1, i] >= width || shape[0, i] >= height || shape[1, i] <= 0 || shape[0, i] <= 0 || field[shape[1, i], shape[0, i]] == 1) return true; return false; }
Kommen wir nun zu den Zahlen. Erstellen Sie ein KeyDown-Ereignis im Konstruktor.
Nach links bewegen:
switch (e.KeyCode){ case Keys.A: for (int i = 0; i < 4; i++) shape[1, i]--;
Ebenso Bewegung nach rechts:
case Keys.D: for (int i = 0; i < 4; i++) shape[1, i]++; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]--; break;
Das Umdrehen der Figur ist etwas komplizierter:
case Keys.W: var shapeT = new int[2, 4]; Array.Copy(shape, shapeT, shape.Length);
Jetzt müssen nur noch ein Tropfen Zahlen hinzugefügt und Linien entfernt werden. All dies wird beim TimerTick-Ereignis geschehen:
private void TickTimer_Tick(object sender, System.EventArgs e){ if (field[8, 3] == 1) Environment.Exit(0);
Und schließlich müssen Sie beim Erstellen des Formulars Folgendes aufrufen:
SetShape();
Endgültiger Code:
using System; using System.Linq; using System.Drawing; using System.Windows.Forms; namespace LittleTetris{ public partial class Form1 : Form{ public const int width = 15, height = 25, k = 15; public int[,] shape = new int[2, 4]; public int[,] field = new int[width, height]; public Bitmap bitfield = new Bitmap(k * (width + 1) + 1, k * (height + 3) + 1); public Graphics gr; public Form1(){ InitializeComponent(); gr = Graphics.FromImage(bitfield); for (int i = 0; i < width; i++) field[i, height - 1] = 1; for (int i = 0; i < height; i++) { field[0, i] = 1; field[width - 1, i] = 1; } SetShape(); } public void FillField(){ gr.Clear(Color.Black); for (int i = 0; i < width; i++) for (int j = 0; j < height; j++) if (field[i, j] == 1){ gr.FillRectangle(Brushes.Green, i * k, j * k, k, k); gr.DrawRectangle(Pens.Black, i * k, j * k, k, k); } for (int i = 0; i < 4; i++){ gr.FillRectangle(Brushes.Red, shape[1, i] * k, shape[0, i] * k, k, k); gr.DrawRectangle(Pens.Black, shape[1, i] * k, shape[0, i] * k, k, k); } FieldPictureBox.Image = bitfield; } private void TickTimer_Tick(object sender, System.EventArgs e){ if (field[8, 3] == 1) Environment.Exit(0); for (int i = 0; i < 4; i++) shape[0, i]++; for (int i = height - 2; i > 2; i--){ var cross = (from t in Enumerable.Range(0, field.GetLength(0)).Select(j => field[j, i]).ToArray() where t == 1 select t).Count(); if (cross == width) for (int k = i; k > 1; k--) for (int l = 1; l < width - 1; l++) field[l, k] = field[l, k - 1];} if (FindMistake()){ for (int i = 0; i < 4; i++) field[shape[1, i], --shape[0, i]]++; SetShape();} FillField(); } private void Form1_KeyDown(object sender, KeyEventArgs e){ switch (e.KeyCode){ case Keys.A: for (int i = 0; i < 4; i++) shape[1, i]--; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]++; break; case Keys.D: for (int i = 0; i < 4; i++) shape[1, i]++; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]--; break; case Keys.W: var shapeT = new int[2, 4]; Array.Copy(shape, shapeT, shape.Length); int maxx = 0, maxy = 0; for (int i = 0; i < 4; i++){ if (shape[0, i] > maxy) maxy = shape[0, i]; if (shape[1, i] > maxx) maxx = shape[1, i]; } for (int i = 0; i < 4; i++) { int temp = shape[0, i]; shape[0, i] = maxy - (maxx - shape[1, i]) - 1; shape[1, i] = maxx - (3 - (maxy - temp)) + 1; } if (FindMistake()) Array.Copy(shapeT, shape, shape.Length); break; } } public void SetShape(){ Random x = new Random(DateTime.Now.Millisecond); switch (x.Next(7)){ case 0: shape = new int[,] { { 2, 3, 4, 5 }, { 8, 8, 8, 8 } }; break; case 1: shape = new int[,] { { 2, 3, 2, 3 }, { 8, 8, 9, 9 } }; break; case 2: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 9 } }; break; case 3: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 7 } }; break; case 4: shape = new int[,] { { 3, 3, 4, 4 }, { 7, 8, 8, 9 } }; break; case 5: shape = new int[,] { { 3, 3, 4, 4 }, { 9, 8, 8, 7 } }; break; case 6: shape = new int[,] { { 3, 4, 4, 4 }, { 8, 7, 8, 9 } }; break; } } public bool FindMistake(){ for (int i = 0; i < 4; i++) if (shape[1, i] >= width || shape[0, i] >= height || shape[1, i] <= 0 || shape[0, i] <= 0 || field[shape[1, i], shape[0, i]] == 1) return true; return false; } } }
Wenn Sie in FindMistake keine Zeilen mit einer schließenden Klammer und einem gemalten Zustand zählen, erhalten Sie 95 Zeilen.
Hier ist ein Screenshot eines laufenden Programms:
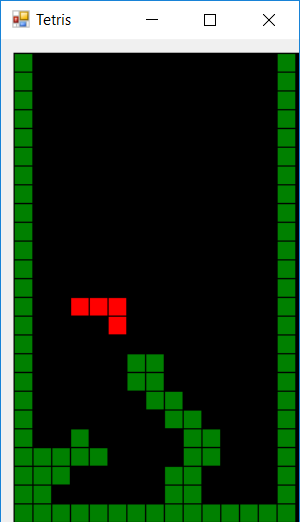