Guten Tag, lieber Leser! Ich, ein Java-Programmierer für Anfänger, konnte mich lange Zeit nicht an die Box2D-Bibliothek gewöhnen. Erstens aufgrund der Tatsache, dass es für C ++ geschrieben wurde und es keine Dokumentation dafür gibt und ich die C-Syntax nicht kenne. Zweitens aufgrund der Tatsache, dass detaillierte Lektionen in dieser Bibliothek nur als Erweiterung von libGDX verfügbar sind. Nach ein paar Wochen harten Kampfes konnte ich endlich verstehen, wie man mit dieser Bibliothek arbeitet, und in diesem Artikel werde ich darüber sprechen (und es zeigen).
Ich arbeite in
Eclipse , der Artikel wird mit dieser Entwicklungsumgebung verbunden. Laden Sie zunächst den
libGDX- Collector herunter und erstellen Sie einen Standard-Build. Wir benötigen eine Desktop-Anwendung mit der Erweiterung Box2D. Gehen Sie dann zu Eclipse, klicken Sie auf Datei → Importieren → Gradle → Gradle-Projekt und geben Sie den Pfad zu Ihrer Baugruppe an.
Hier ist ein Bild, das zeigt, wie meins aussieht. Ich habe das Utils-Paket mit der Constants-Klasse zum Core-Ordner hinzugefügt, der nur eine Konstante enthält - die Anzahl der Pixel pro Meter. Dies ist so, dass die Welt nicht gigantisch ist.
Hier ist der Code für die DesktopLauncher-Klasse von com.mygdx.game.desktop:
Fügen Sie diesen Code in die Klasse ein und vergessen Sie ihnpackage com.mygdx.game.desktop; import com.badlogic.gdx.backends.lwjgl.LwjglApplication; import com.badlogic.gdx.backends.lwjgl.LwjglApplicationConfiguration; import com.mygdx.game.MyGdxGame; public class DesktopLauncher { public static void main(String[] arg) { LwjglApplicationConfiguration config = new LwjglApplicationConfiguration();
Es wird viel Code geben, also werde ich ihn in Spoiler einschließen. Nur die MyGdxGame-Klasse aus dem com.mygdx.game-Paket wird geändert.
Versuchen wir also, in unserer Anwendung eine ziemlich einfache Sache zu machen. Der Weg des Balls kracht von den Brettern gegen die Wand und sie zerstreuen sich. So etwas wie das:
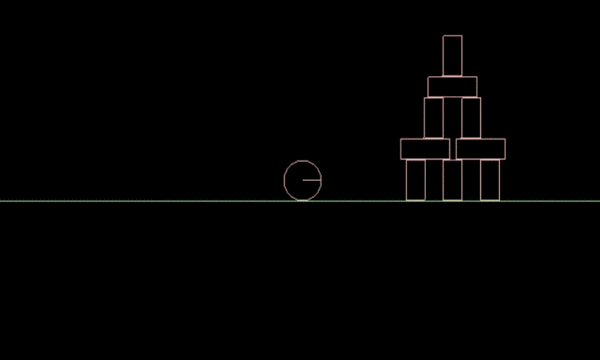
Idee 1. Bowling package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.Input.Keys; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr;
Es ist großartig geworden, oder? Durch Ändern mehrerer Parameter können Sie genau das erreichen, was Sie brauchen! Und schreiben Sie nicht so viele Codezeilen.
Aber jetzt möchte ich einen elastischen Schlag sehen. Und lass es mehr Objekte auf der Bühne geben. Wir erhalten folgendes Ergebnis:
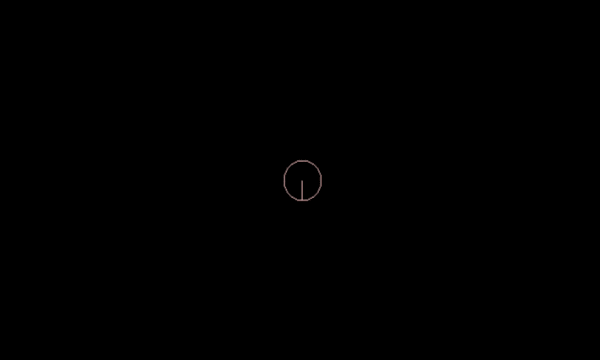
Und hier ist der Code: package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.physics.box2d.FixtureDef; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer();
Das Erstellen eines Warenkorbs in Box2D ist nicht einfach. Es ist notwendig, die Körper so zu binden, dass sie sich als Ganzes bewegen. Das folgende GIF zeigt nur das Wesentliche.
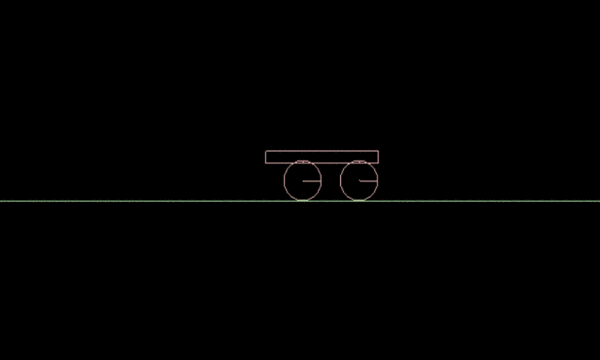
Wie kam es dazu? package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.Input; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body ball1; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer();
Wenn Sie sich den Code ansehen, werden Sie feststellen, dass dies kein echter Wagen ist, sondern ein Stock auf Rädern. Gehen Sie Enthusiasten! Auf Youtube gibt es ein
Video, in dem ein Viertakt-ICE in Box2D hergestellt wurde. Sind wir schlimmer Warten Sie in den Kommentaren auf Ihren Erfolg!
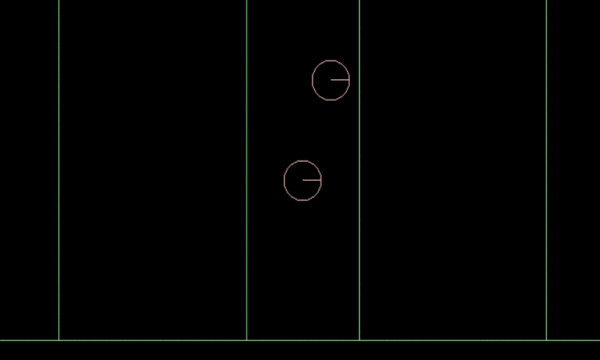
Noch mehr Zusammenstöße! package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.FixtureDef; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body ball1; private Body ball2; private Body ball3; private Body ball4; private Body ball5; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer();
Und das letzte GIF für heute. Die schiefe Ebene ist uns aus dem Physikunterricht bekannt. Dieser Code beschreibt, wie Sie eine komplexere Form (theoretisch beliebig) erhalten können, so dass sich unbegrenzte Möglichkeiten für die Spielphysik eröffnen.

Geneigte Ebene public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body floor; private Body wall; private Body plos; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer(); ball = createPlayer(); floor = createfloor(); wall = createwall(430, 170); wall = createwall(-430, 170); plos = createplos(); } public void render() { update(Gdx.graphics.getDeltaTime()); Gdx.gl.glClearColor(0, 0, 0, 1); Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT); b2dr.render(world, camera.combined.scl(Constants.PPM)); } public void resize(int width, int height) { camera.setToOrtho(false, width / 2, height / 2); } public void dispose() { world.dispose(); b2dr.dispose(); } public void update(float delta) { world.step(1 / 60f, 6, 2); cameraUpdate(delta); } public void cameraUpdate(float delta) { Vector3 position = camera.position; position.x = ball.getPosition().x * Constants.PPM; position.y = ball.getPosition().y * Constants.PPM; camera.position.set(position); camera.update(); } public Body createPlayer() { Body pBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.DynamicBody; def.position.set(30 / Constants.PPM, 190 / Constants.PPM); def.fixedRotation = false; pBody = world.createBody(def); CircleShape shape = new CircleShape(); shape.setRadius(10 / Constants.PPM); pBody.createFixture(shape, 1.0f); def.bullet = true; shape.dispose(); return pBody; } public Body createfloor() { Body fBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.StaticBody; def.position.set(0, 0); def.fixedRotation = true; fBody = world.createBody(def); PolygonShape shape = new PolygonShape(); shape.setAsBox(480 / Constants.PPM, 70 / Constants.PPM); fBody.createFixture(shape, 0.001f); shape.dispose(); return fBody; } public Body createwall(int xo, int yo) { Body fBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.StaticBody; def.position.set(xo / Constants.PPM, yo / Constants.PPM); def.fixedRotation = true; fBody = world.createBody(def); PolygonShape shape = new PolygonShape(); shape.setAsBox(50 / Constants.PPM, 100 / Constants.PPM); fBody.createFixture(shape, 0.001f); shape.dispose(); return fBody; } public Body createplos() { Vector2[] vertices = new Vector2[3]; vertices[0] = new Vector2(0f , -0.6f ); vertices[1] = new Vector2(1f , -0.6f ); vertices[2] = new Vector2(1f , 1f); PolygonShape shape = new PolygonShape(); Body fBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.StaticBody; def.position.set(20 / Constants.PPM, 90 / Constants.PPM); def.fixedRotation = true; fBody = world.createBody(def); shape.set(vertices); fBody.createFixture(shape, 0.001f); shape.dispose(); return fBody; } }
Ich hoffe, dass Sie dank dieses Codes die Grundlagen von Box2D verstehen und äußerst hervorragende Anwendungen entstehen! Vielen Dank für das Lesen bis zum Ende! Ich werde versuchen, alle Fragen in den Kommentaren zu beantworten!