Como debería ser un concierto decente, tengo una estación meteorológica, que ensamblé yo mismo a partir de la pantalla DHT22, Raspberry Pi y Nokia, esta es una solución de potencia constante que transmite datos a través de Ethernet.
Pero ahora necesitaba una estación meteorológica móvil en el balcón, inalámbrica y con pilas.
Como módulo de radio utilicé una placa Z-Wave Z-Uno, como sensor de temperatura y humedad decidí probar Sensirion SHT20 trabajando en I2C.
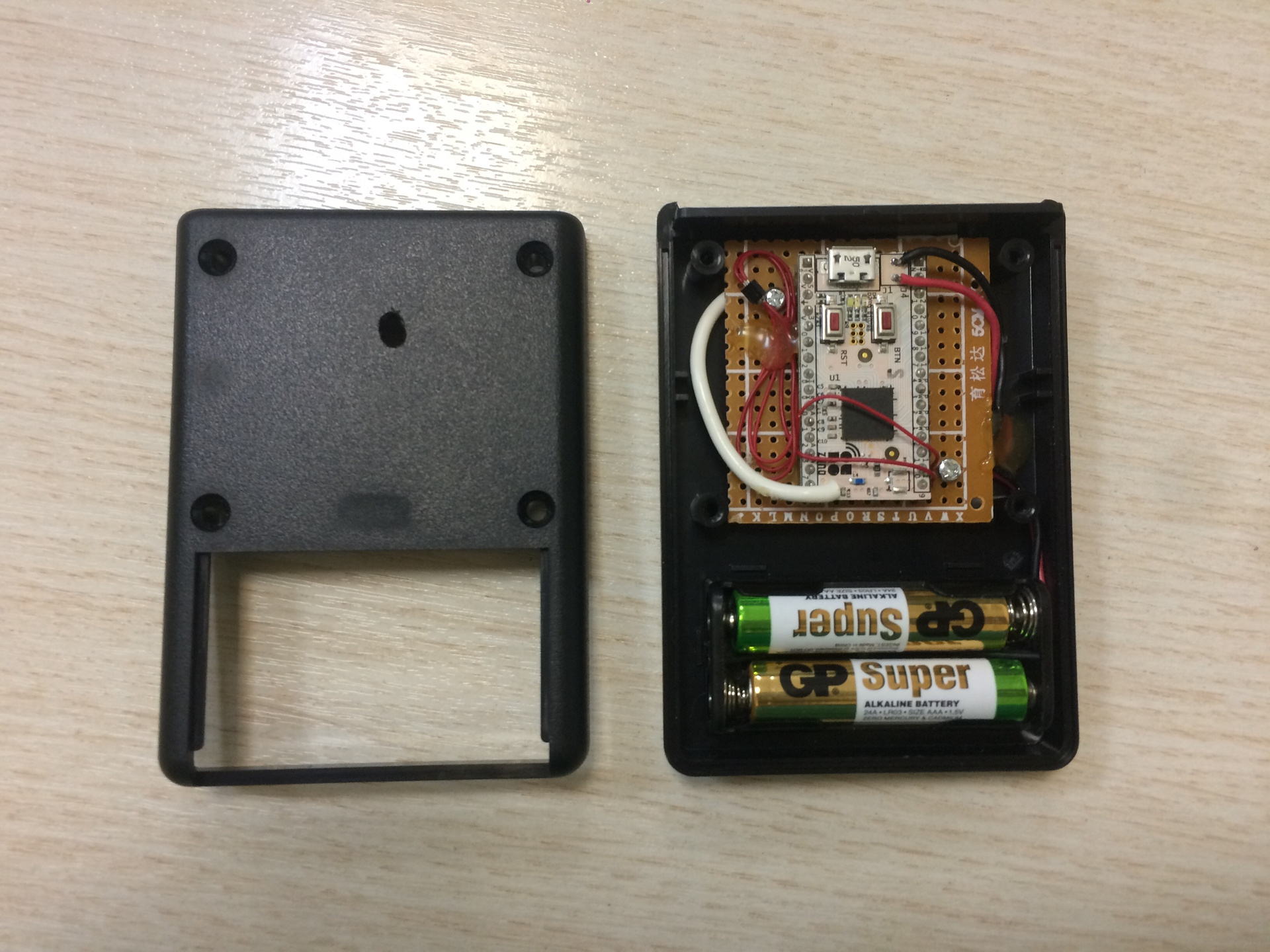
Materiales y precios:
Z-Uno se actualiza constantemente, se agrega soporte para nuevos periféricos y bibliotecas. Por el momento, la versión actual del firmware es 2.0.9 y ha aparecido la compatibilidad con los siguientes dispositivos:
- Sensor de temperatura DS18B20 de 1 cable
- Sensor de temperatura y humedad DHT22 && DHT11
- Lector de tarjetas RFID de 125Khz
- Sensor de presión y temperatura I2C BMP180
- y otros dispositivos, ejemplos aquí son z-uno.z-wave.me/examples
El sensor Sensirion SHT20 que funciona en el bus I2C no estaba en la lista de los admitidos, por lo que tuve que escribir mi propia implementación para Z-Uno. La sintaxis del boceto para Z-Uno es lo más similar posible a Arduino, todo el boceto cabe en 35 líneas:

Boceto para Z-Uno sobre cómo trabajar con un sensor de temperatura y humedad Sensirion SHT20#include <Wire.h> #include "SHT2x.h" int16_t temperature; uint8_t humidity; ZUNO_SETUP_SLEEPING_MODE(ZUNO_SLEEPING_MODE_SLEEPING); #define ZUNO_CHANNEL_TEMPERATURE 1 #define ZUNO_CHANNEL_HUMIDITY 2 ZUNO_SETUP_CHANNELS( ZUNO_SENSOR_MULTILEVEL_TEMPERATURE(getterTemp), ZUNO_SENSOR_MULTILEVEL_HUMIDITY(getterHumid) ); void setup() { Wire.begin(); } void loop() { temperature = SHT2x.GetTemperature(); zunoSendReport(ZUNO_CHANNEL_TEMPERATURE); humidity = SHT2x.GetHumidity(); zunoSendReport(ZUNO_CHANNEL_HUMIDITY); zunoSendDeviceToSleep(); } byte getterTemp(void) { return temperature; } byte getterHumid(void) { return humidity; }
SHT2x.cpp modificado para Z-Uno SHT2x.h #ifndef SHT2X_H #define SHT2X_H
De las cosas especiales, en el boceto solo hay una línea que pone a Z-Uno en modo de suspensión:
ZUNO_SETUP_SLEEPING_MODE(ZUNO_SLEEPING_MODE_SLEEPING)
Y configurar a qué canal enviar la temperatura y en qué humedad:
ZUNO_SETUP_CHANNELS( ZUNO_SENSOR_MULTILEVEL_TEMPERATURE(getterTemp), ZUNO_SENSOR_MULTILEVEL_HUMIDITY(getterHumid) )
El resto es código Arduino normal.
Sensirion SHT20 funciona en I2C y está conectado por 2 cables. De manera predeterminada, en la biblioteca Wire.h para I2C, se definen los pines 9 y 10.
La información del sensor se puede ver en el teléfono y se utiliza en la automatización para controlar el humidificador y el aire acondicionado.
Los datos se actualizan cada 12 minutos, en este modo, el sensor debe funcionar durante más de 2 años con un conjunto de baterías.
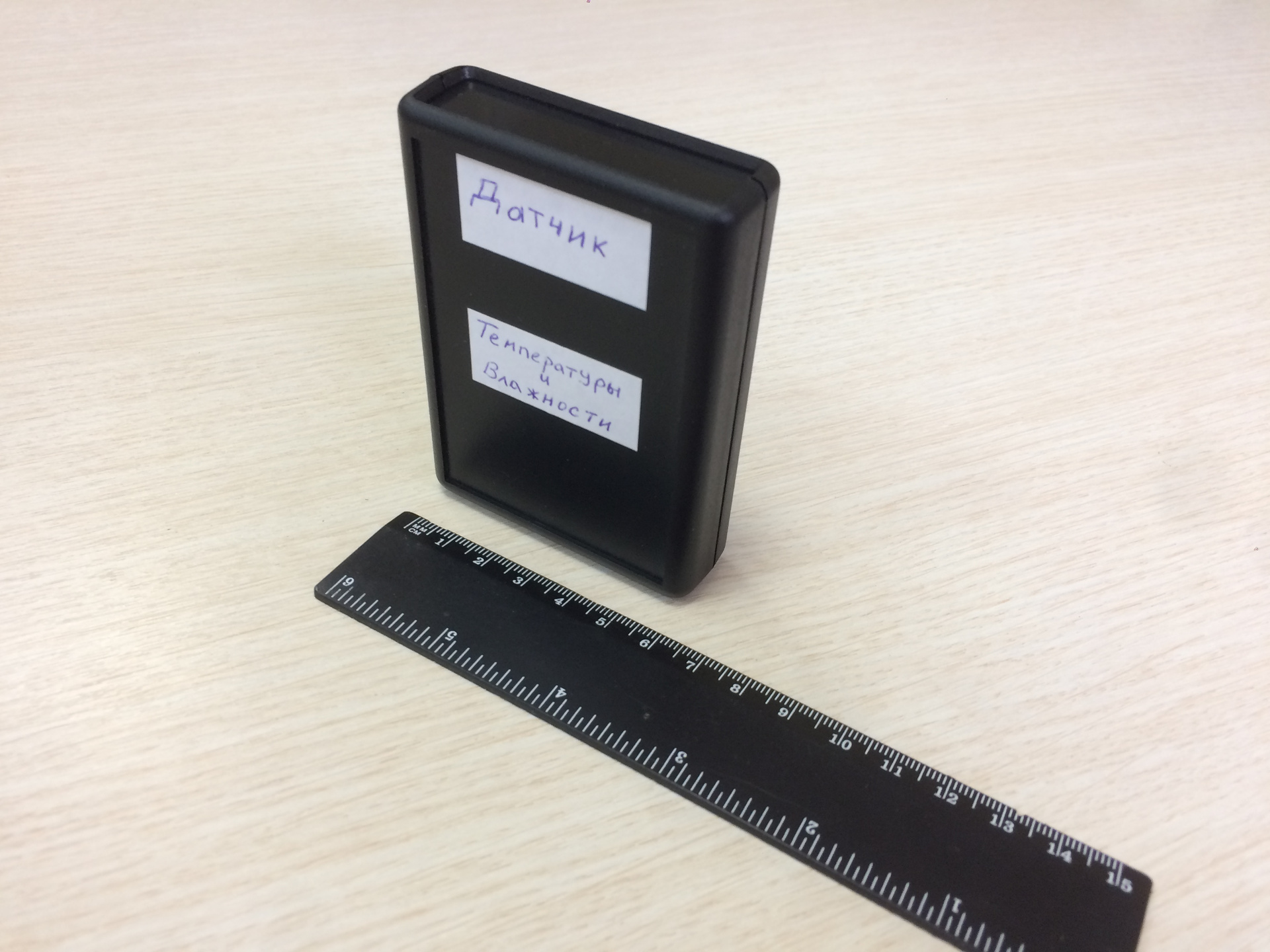
El edificio Gint G517B 90 x 60 x 20 cm fue especialmente elegido con un lugar debajo del compartimento de la batería, todo encaja perfectamente.
El desarrollo del sensor de temperatura y humedad Z-Wave tomó aproximadamente 2 horas y 3746 p. ¡También puede agregar una pantalla de tinta electrónica y colgarla en la pared!