UPD
Enlace a github.
Recientemente, se me ocurrió una idea: escribir un juego simple con un número mínimo de líneas. Mi elección recayó en Tetris. En este artículo describiré mi código.
Para empezar, vale la pena señalar que en mi implementación incluí solo las características básicas:
- movimiento de figuras izquierda / derecha;
- figuras que caen;
- rotación de figuras;
- eliminar figuras rellenas;
- El final del juego.
Entonces, primero agregue un PictureBox al formulario y cree un temporizador.
También para el juego necesitarás:
public const int width = 15, height = 25, k = 15;
Rellene el campo alrededor de los bordes:
for (int i = 0; i < width; i++) field[i, height - 1] = 1; for (int i = 0; i < height; i++) { field[0, i] = 1; field[width - 1, i] = 1; }
Rellena la figura:
public void SetShape(){ Random x = new Random(DateTime.Now.Millisecond); switch (x.Next(7)){
El procedimiento que dibuja el "vidrio" en PictureBox:
public void FillField(){ gr.Clear(Color.Black);
Primero muevo las figuras y luego verifico si esta opción es posible o no. Si en algún lugar hay un error (la figura está fuera del campo o superpuesta a la figura que ya está en el campo), la figura vuelve a su lugar original. Para hacer esto, escribí una función que devuelve verdadero si se encontró un error en el campo o falso si no hay error:
public bool FindMistake(){ for (int i = 0; i < 4; i++) if (shape[1, i] >= width || shape[0, i] >= height || shape[1, i] <= 0 || shape[0, i] <= 0 || field[shape[1, i], shape[0, i]] == 1) return true; return false; }
Ahora pasemos a las figuras. Cree un evento KeyDown en el constructor.
Mover a la izquierda:
switch (e.KeyCode){ case Keys.A: for (int i = 0; i < 4; i++) shape[1, i]--;
Del mismo modo, movimiento hacia la derecha:
case Keys.D: for (int i = 0; i < 4; i++) shape[1, i]++; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]--; break;
El giro de la figura es un poco más complicado:
case Keys.W: var shapeT = new int[2, 4]; Array.Copy(shape, shapeT, shape.Length);
Ahora solo queda agregar una gota de figuras y eliminar líneas. Todo esto sucederá en el evento TimerTick:
private void TickTimer_Tick(object sender, System.EventArgs e){ if (field[8, 3] == 1) Environment.Exit(0);
Y finalmente, al crear el formulario, debe llamar a:
SetShape();
Código final:
using System; using System.Linq; using System.Drawing; using System.Windows.Forms; namespace LittleTetris{ public partial class Form1 : Form{ public const int width = 15, height = 25, k = 15; public int[,] shape = new int[2, 4]; public int[,] field = new int[width, height]; public Bitmap bitfield = new Bitmap(k * (width + 1) + 1, k * (height + 3) + 1); public Graphics gr; public Form1(){ InitializeComponent(); gr = Graphics.FromImage(bitfield); for (int i = 0; i < width; i++) field[i, height - 1] = 1; for (int i = 0; i < height; i++) { field[0, i] = 1; field[width - 1, i] = 1; } SetShape(); } public void FillField(){ gr.Clear(Color.Black); for (int i = 0; i < width; i++) for (int j = 0; j < height; j++) if (field[i, j] == 1){ gr.FillRectangle(Brushes.Green, i * k, j * k, k, k); gr.DrawRectangle(Pens.Black, i * k, j * k, k, k); } for (int i = 0; i < 4; i++){ gr.FillRectangle(Brushes.Red, shape[1, i] * k, shape[0, i] * k, k, k); gr.DrawRectangle(Pens.Black, shape[1, i] * k, shape[0, i] * k, k, k); } FieldPictureBox.Image = bitfield; } private void TickTimer_Tick(object sender, System.EventArgs e){ if (field[8, 3] == 1) Environment.Exit(0); for (int i = 0; i < 4; i++) shape[0, i]++; for (int i = height - 2; i > 2; i--){ var cross = (from t in Enumerable.Range(0, field.GetLength(0)).Select(j => field[j, i]).ToArray() where t == 1 select t).Count(); if (cross == width) for (int k = i; k > 1; k--) for (int l = 1; l < width - 1; l++) field[l, k] = field[l, k - 1];} if (FindMistake()){ for (int i = 0; i < 4; i++) field[shape[1, i], --shape[0, i]]++; SetShape();} FillField(); } private void Form1_KeyDown(object sender, KeyEventArgs e){ switch (e.KeyCode){ case Keys.A: for (int i = 0; i < 4; i++) shape[1, i]--; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]++; break; case Keys.D: for (int i = 0; i < 4; i++) shape[1, i]++; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]--; break; case Keys.W: var shapeT = new int[2, 4]; Array.Copy(shape, shapeT, shape.Length); int maxx = 0, maxy = 0; for (int i = 0; i < 4; i++){ if (shape[0, i] > maxy) maxy = shape[0, i]; if (shape[1, i] > maxx) maxx = shape[1, i]; } for (int i = 0; i < 4; i++) { int temp = shape[0, i]; shape[0, i] = maxy - (maxx - shape[1, i]) - 1; shape[1, i] = maxx - (3 - (maxy - temp)) + 1; } if (FindMistake()) Array.Copy(shapeT, shape, shape.Length); break; } } public void SetShape(){ Random x = new Random(DateTime.Now.Millisecond); switch (x.Next(7)){ case 0: shape = new int[,] { { 2, 3, 4, 5 }, { 8, 8, 8, 8 } }; break; case 1: shape = new int[,] { { 2, 3, 2, 3 }, { 8, 8, 9, 9 } }; break; case 2: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 9 } }; break; case 3: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 7 } }; break; case 4: shape = new int[,] { { 3, 3, 4, 4 }, { 7, 8, 8, 9 } }; break; case 5: shape = new int[,] { { 3, 3, 4, 4 }, { 9, 8, 8, 7 } }; break; case 6: shape = new int[,] { { 3, 4, 4, 4 }, { 8, 7, 8, 9 } }; break; } } public bool FindMistake(){ for (int i = 0; i < 4; i++) if (shape[1, i] >= width || shape[0, i] >= height || shape[1, i] <= 0 || shape[0, i] <= 0 || field[shape[1, i], shape[0, i]] == 1) return true; return false; } } }
Como resultado, si no cuenta líneas con un corchete de cierre y una condición pintada en FindMistake, obtendrá 95 líneas.
Aquí hay una captura de pantalla de un programa en ejecución:
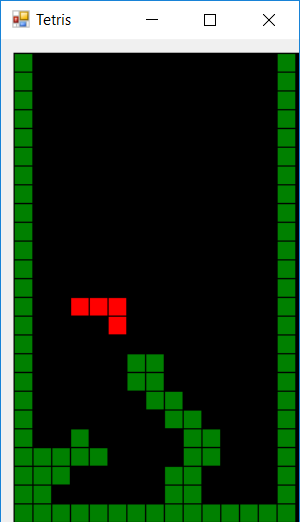