Comme il convient, j'ai une station météo que j'ai moi-même montée à partir du DHT22, du Raspberry Pi et de l'écran Nokia, c'est une solution d'alimentation constante qui transmet des données via Ethernet.
Mais maintenant, j'avais besoin d'une station météo mobile sur le balcon, sans fil et alimentée par batterie.
En tant que module radio, j'ai utilisé une carte Z-Wave Z-Uno, comme capteur de température et d'humidité, j'ai décidé d'essayer Sensirion SHT20 en travaillant sur I2C.
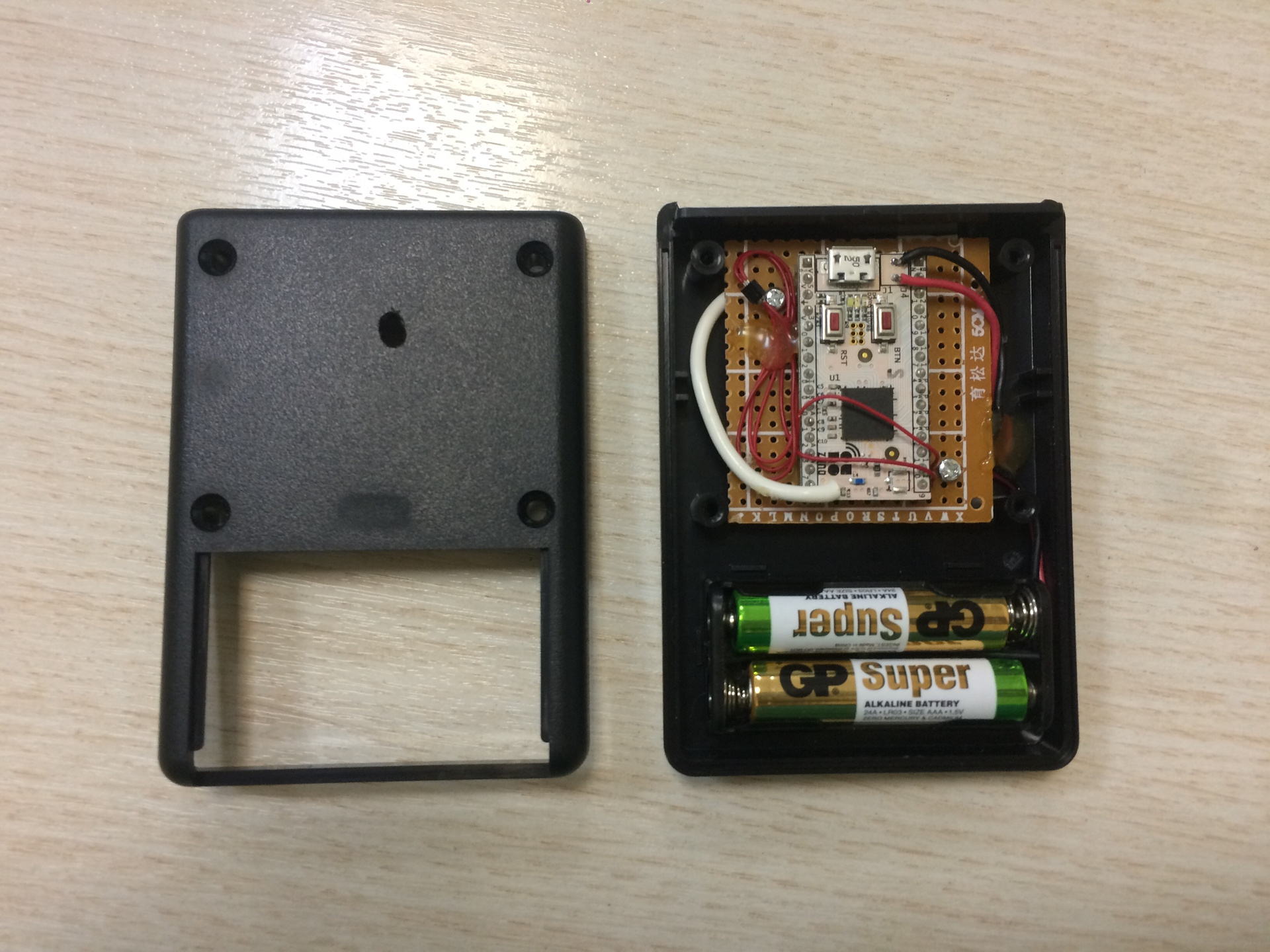
Matériaux et prix:
Z-Uno est constamment mis à jour, la prise en charge de nouveaux périphériques et bibliothèques est ajoutée. À l'heure actuelle, la version actuelle du micrologiciel est 2.0.9 et la prise en charge des appareils suivants y est apparue:
- Capteur de température DS18B20 à 1 fil
- Capteur de température et d'humidité DHT22 && DHT11
- Lecteur de carte RFID 125Khz
- Capteur de pression et de température I2C BMP180
- et d'autres appareils, des exemples ici sont z-uno.z-wave.me/examples
Le capteur Sensirion SHT20 fonctionnant sur le bus I2C n'était pas dans la liste des capteurs pris en charge, j'ai donc dû écrire ma propre implémentation pour Z-Uno. La syntaxe d'esquisse pour Z-Uno est aussi similaire à Arduino que possible, l'esquisse entière tient en 35 lignes:

Esquisse pour Z-Uno sur l'utilisation d'un capteur de température et d'humidité Sensirion SHT20#include <Wire.h> #include "SHT2x.h" int16_t temperature; uint8_t humidity; ZUNO_SETUP_SLEEPING_MODE(ZUNO_SLEEPING_MODE_SLEEPING); #define ZUNO_CHANNEL_TEMPERATURE 1 #define ZUNO_CHANNEL_HUMIDITY 2 ZUNO_SETUP_CHANNELS( ZUNO_SENSOR_MULTILEVEL_TEMPERATURE(getterTemp), ZUNO_SENSOR_MULTILEVEL_HUMIDITY(getterHumid) ); void setup() { Wire.begin(); } void loop() { temperature = SHT2x.GetTemperature(); zunoSendReport(ZUNO_CHANNEL_TEMPERATURE); humidity = SHT2x.GetHumidity(); zunoSendReport(ZUNO_CHANNEL_HUMIDITY); zunoSendDeviceToSleep(); } byte getterTemp(void) { return temperature; } byte getterHumid(void) { return humidity; }
SHT2x.cpp modifié pour Z-Uno SHT2x.h #ifndef SHT2X_H #define SHT2X_H
Parmi les choses spéciales, dans l'esquisse, il n'y a qu'une ligne qui met Z-Uno en mode veille:
ZUNO_SETUP_SLEEPING_MODE(ZUNO_SLEEPING_MODE_SLEEPING)
Et configurer quel canal envoyer la température et dans quelle humidité:
ZUNO_SETUP_CHANNELS( ZUNO_SENSOR_MULTILEVEL_TEMPERATURE(getterTemp), ZUNO_SENSOR_MULTILEVEL_HUMIDITY(getterHumid) )
Le reste est du code Arduino normal.
Sensirion SHT20 fonctionne sur I2C et est connecté par 2 fils. Par défaut, dans la bibliothèque Wire.h pour I2C, les broches 9 et 10 sont définies.
Les informations du capteur peuvent être consultées sur le téléphone et sont utilisées dans l'automatisation pour contrôler l'humidificateur et la climatisation.
Les données sont mises à jour toutes les 12 minutes, dans ce mode, le capteur doit fonctionner pendant plus de 2 ans à partir d'un jeu de piles.
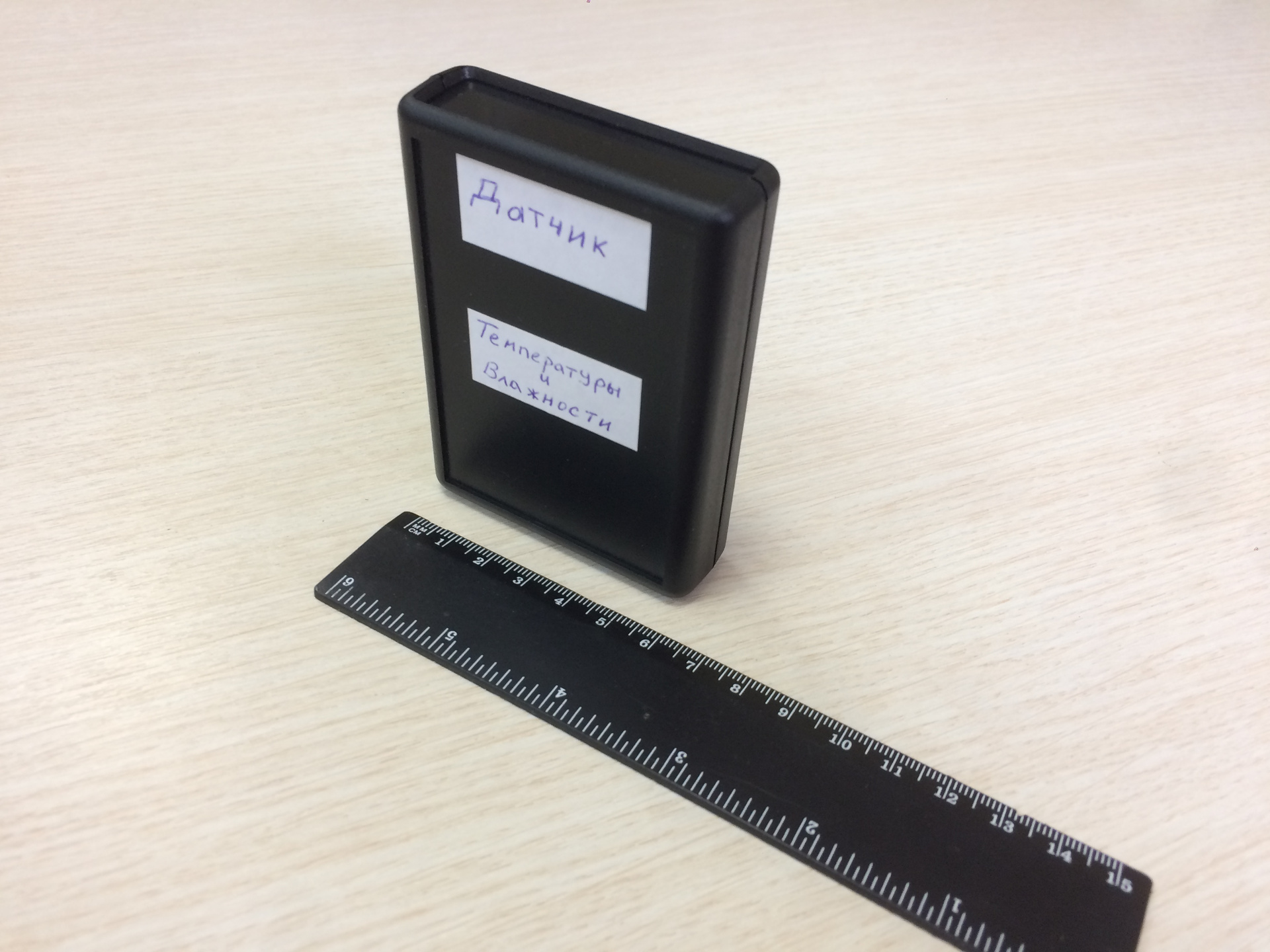
Le bâtiment Gint G517B 90 x 60 x 20 cm a été spécialement choisi avec une place sous le compartiment batterie, tout s'intègre parfaitement.
Le développement du capteur de température et d'humidité Z-Wave a pris environ 2 heures et 3746 p. Vous pouvez également ajouter un écran E-Ink et l'accrocher au mur!