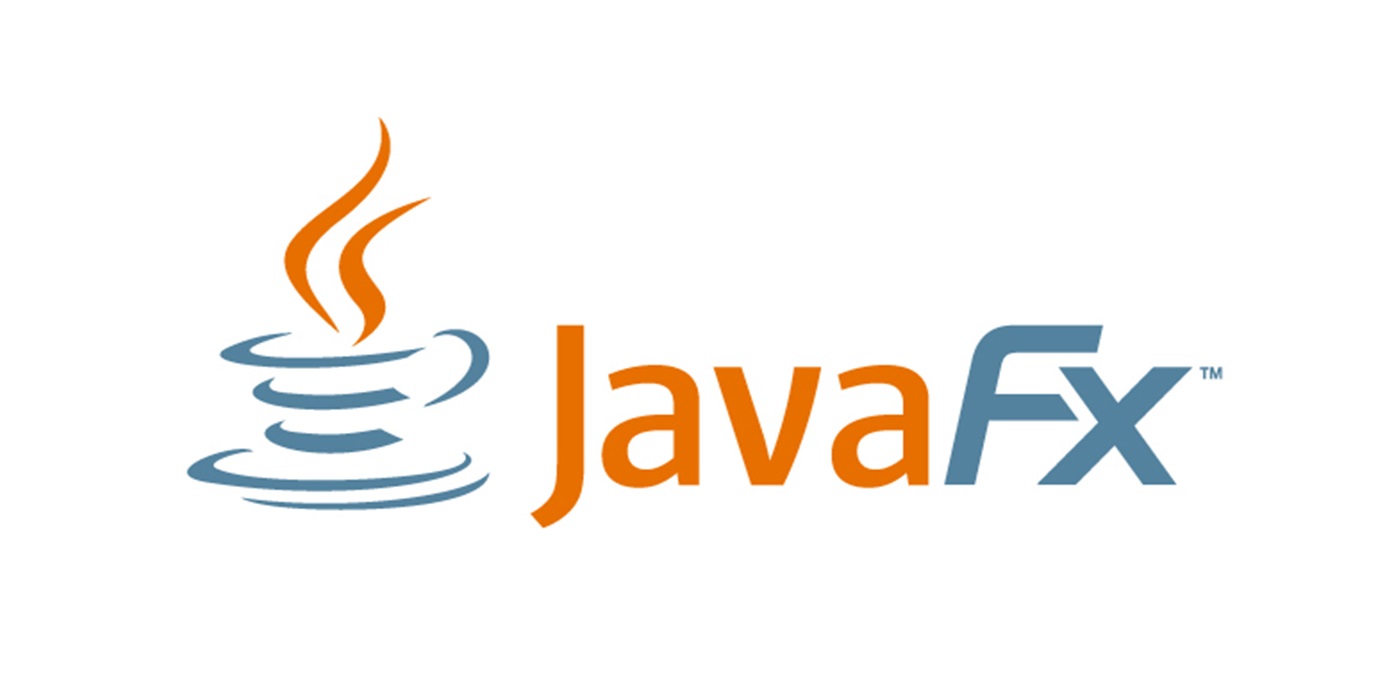
Oi
Até recentemente, meu conhecimento em criar qualquer tipo de interface gráfica era zero. Portanto, foi decidido mexer levemente a Internet e criar uma janela com alguns botões; quando clicados, algo acontecerá. Talvez neste texto alguém encontre respostas para as perguntas que surgiram uma vez; ao mesmo tempo, espero críticas sérias e competentes.
O que vai acontecer?
Como meu objetivo é lidar com o JavaFX, preciso criar algum tipo de função em nossa janela. A idéia é a seguinte: criar um conjunto de pessoas (suponha que elas trabalhem em uma empresa), para cada uma das quais saberemos o nome, idade, salário e estado civil. Nossa janela, em primeiro lugar, exibirá informações sobre cada um deles, em segundo lugar, alterará o salário de um funcionário, em terceiro lugar, poderá adicionar um novo funcionário e, finalmente, em quarto lugar, poderá filtrar os funcionários, dependendo a partir dos valores de seus atributos. O resultado deve ser algo como isto:

Introdução
Primeiro, criamos a classe principal da empresa, de onde o aplicativo será iniciado:
package company; public class Company { public static void main(String[] args) { } }
Nesta classe,
expandiremos os recursos da classe Application. A partir dele, precisamos principalmente do método start (), no qual a ação principal ocorrerá (pularemos os erros que ocorrem nesse método; em outras palavras, se algo der errado, nossa janela não fechará, mas os erros do StackTrace aparecerão no console) :
package company; import javafx.application.Application; import javafx.stage.Stage; public class Company extends Application { public void start(Stage primaryStage) throws Exception { } public static void main(String[] args) { launch(args); } }
Ótimo. Para trabalhar com representantes dos funcionários da empresa, crie a classe User: objetos desta classe e atuará como funcionários.
Classe de usuário package company; public class User { User(String name, int age, int salary, boolean married) { this.name = name; this.age = age; this.salary = salary; this.married = married; } public void changeSalary(int x) { salary = (salary + x <= 0)? 0: salary + x; } public String toString() { return name; } public String getAge() { return String.valueOf(age); } public String getSalary() { return String.valueOf(salary); } public String getMarried() { return (married)? ("married"):("single"); } String name; int age; int salary; boolean married; }
Criar
Até o momento, quando o aplicativo é iniciado, nada acontece para nós. Para que pelo menos uma janela apareça, você deve adicioná-la. Nós vamos fazer isso.
package company; import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; public class Company extends Application { public void start(Stage primaryStage) throws Exception { Scene scene = new Scene(root, WIDTH, HEIGHT); primaryStage.setTitle("Company"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } Group root = new Group(); final private int WIDTH = 1000; final private int HEIGHT = 600; }
O que acabamos de fazer: adicionamos uma cena de tamanho
, deu a ela o nome "Empresa". Há um grupo
raiz em nossa cena, que é essencialmente um "contêiner" que conterá todos os botões.
Adicione uma lista suspensa com nossos funcionários. Crie uma HBox, na qual colocamos nossa lista suspensa combobox userBox e um botão que exibirá informações sobre o funcionário (colocaremos a HBox na VBox):
package company; import java.util.ArrayList; import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.ComboBox; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.text.Text; import javafx.stage.Stage; public class Company extends Application { public void start(Stage primaryStage) throws Exception { users.add(new User("Alice", 20, 150, false)); users.add(new User("Bob", 34, 300, true)); users.add(new User("Peter", 18, 100, false)); users.add(new User("Kate", 38, 300, true)); users.add(new User("Steve", 31, 250, true)); users.add(new User("Alan", 62, 500, true)); users.add(new User("Julia", 33, 320, true)); users.add(new User("Patric", 37, 300, true)); users.add(new User("Alexander", 34, 280, true)); users.add(new User("George", 28, 180, true)); users.add(new User("Mary", 22, 190, false)); userBox.getItems().addAll(users); root.getChildren().add(strings); strings.setPadding(new Insets(10, 30, 10, 30)); strings.setSpacing(20); strings.getChildren().add(new Text("Select the user")); strings.getChildren().add(buttonBox); buttonBox.setSpacing(10); buttonBox.getChildren().add(userBox); Scene scene = new Scene(root, WIDTH, HEIGHT); primaryStage.setTitle("Company"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } Group root = new Group(); VBox strings = new VBox(); HBox buttonBox = new HBox(); ComboBox<User> userBox = new ComboBox<>(); final private int WIDTH = 1000; final private int HEIGHT = 600; private ArrayList<User> users = new ArrayList<>(); }

O que são VBox, HBox e ComboBox
HBox é um conjunto de botões, textos, campos de entrada, cada objeto organizado horizontalmente em sequência. Semelhante, o VBox é o mesmo, mas armazena seus objetos (filhos) verticalmente. Usaremos o seguinte esquema: crie uma VBox na qual colocaremos vários HBox'ov, em cada um dos quais colocaremos uma sequência de botões e campos.
ComboBox é apenas uma lista suspensa em si.
Agora queremos criar um botão que exibirá informações sobre o funcionário selecionado. Adicione o próprio botão (Button) e o campo de texto (TextField), no qual o texto será exibido. Nós adicionamos esses dois objetos ao HBox:
package company; public class Company extends Application { public void start(Stage primaryStage) throws Exception { buttonBox.setSpacing(10); buttonBox.getChildren().add(userBox); buttonBox.getChildren().add(buttonGetInfo); buttonBox.getChildren().add(textInfo); } Button buttonGetInfo = new Button("Info"); Text textInfo = new Text(); }

Mas até agora este botão não faz nada. Para que ela faça algo, ela deve receber uma ação:
buttonGetInfo.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { User u = (User) userBox.getSelectionModel().getSelectedItem(); if (u != null) { textInfo.setText("Age is " + u.getAge() + ", " + "Salary is " + u.getSalary() + ", " + "Relationship: " + u.getMarried()); } else { textInfo.setText("User not selected"); } } });

Ações adicionais
Exatamente da mesma maneira, adicionamos mais dois HBox: no segundo haverá uma alteração no salário, no terceiro - a adição de um novo funcionário. Devido ao fato de a lógica aqui ser exatamente a mesma, pulamos a explicação desses pontos e imediatamente mostramos o resultado:

Código package company; import java.util.ArrayList; import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.Insets; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.ComboBox; import javafx.scene.control.TextField; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.text.Text; import javafx.stage.Stage; public class Company extends Application { public void start(Stage primaryStage) throws Exception { users.add(new User("Alice", 20, 150, false)); users.add(new User("Bob", 34, 300, true)); users.add(new User("Peter", 18, 100, false)); users.add(new User("Kate", 38, 300, true)); users.add(new User("Steve", 31, 250, true)); users.add(new User("Alan", 62, 500, true)); users.add(new User("Julia", 33, 320, true)); users.add(new User("Patric", 37, 300, true)); users.add(new User("Alexander", 34, 280, true)); users.add(new User("George", 28, 180, true)); users.add(new User("Mary", 22, 190, false)); userBox.getItems().addAll(users); root.getChildren().add(strings); strings.setPadding(new Insets(10, 30, 10, 30)); strings.setSpacing(20); strings.getChildren().add(new Text("Select the user")); strings.getChildren().add(buttonBox); strings.getChildren().add(new Text("Change the salary")); strings.getChildren().add(changeSalaryBox); strings.getChildren().add(new Text("Add new User")); strings.getChildren().add(addUserBox); buttonBox.setSpacing(10); buttonBox.getChildren().add(userBox); buttonBox.getChildren().add(buttonGetInfo); buttonBox.getChildren().add(textInfo); changeSalaryBox.setSpacing(10); changeSalaryBox.getChildren().add(buttonChangeSalary); changeSalaryBox.getChildren().add(howMuchChange); addUserBox.setSpacing(10); addUserBox.getChildren().add(new Text("Name: ")); addUserBox.getChildren().add(name); addUserBox.getChildren().add(new Text("Age: ")); addUserBox.getChildren().add(age); addUserBox.getChildren().add(new Text("Salary: ")); addUserBox.getChildren().add(salary); addUserBox.getChildren().add(new Text("Married: ")); addUserBox.getChildren().add(married); addUserBox.getChildren().add(buttonAddUser); buttonGetInfo.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { User u = (User) userBox.getSelectionModel().getSelectedItem(); if (u != null) { textInfo.setText("Age is " + u.getAge() + ", " + "Salary is " + u.getSalary() + ", " + "Relationship: " + u.getMarried()); } else { textInfo.setText("User not selected"); } } }); buttonChangeSalary.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { User u = (User) userBox.getSelectionModel().getSelectedItem(); if(u != null) { u.changeSalary(Integer.parseInt(howMuchChange.getText())); textInfo.setText("Age is " + u.getAge() + ", " + "Salary is " + u.getSalary() + ", " + "Relationshp: " + u.getMarried()); howMuchChange.clear(); } } }); buttonAddUser.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { String m = married.getText(); boolean mm = (m.equals("married"))? true:false; User u = new User(name.getText(), Integer.parseInt(age.getText()), Integer.parseInt(salary.getText()), mm); users.add(u); userBox.getItems().addAll(u); name.clear(); age.clear(); salary.clear(); married.clear(); } }); Scene scene = new Scene(root, WIDTH, HEIGHT); primaryStage.setTitle("Company"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } Group root = new Group(); VBox strings = new VBox(); HBox buttonBox = new HBox(); ComboBox<User> userBox = new ComboBox<>(); Button buttonGetInfo = new Button("Info"); Text textInfo = new Text(); HBox changeSalaryBox = new HBox(); Button buttonChangeSalary = new Button("Change salary"); TextField howMuchChange = new TextField(); HBox addUserBox = new HBox(); Button buttonAddUser = new Button("Add User"); TextField name = new TextField(); TextField age = new TextField(); TextField salary = new TextField(); TextField married = new TextField(); final private int WIDTH = 1000; final private int HEIGHT = 600; private ArrayList<User> users = new ArrayList<>(); }
Filtros
Agora adicione a "filtragem" dos funcionários por características. Novamente, adicionaremos listas de filtros usando o ComboBox usando a mesma lógica.
package company; public class Company extends Application { public void start(Stage primaryStage) throws Exception { ageFilterBox.getItems().addAll( "no matter", "over 20", "over 30", "over 40" ); salaryFilterBox.getItems().addAll( "no matter", "over 150", "over 250", "over 500" ); relationshipFilterBox.getItems().addAll( "no matter", "married", "single" ); strings.getChildren().add(filters); strings.getChildren().add(resultFilter); filters.setSpacing(10); filters.getChildren().add(new Text("Age")); filters.getChildren().add(ageFilterBox); filters.getChildren().add(new Text("Salary")); filters.getChildren().add(salaryFilterBox); filters.getChildren().add(new Text("Relationship")); filters.getChildren().add(relationshipFilterBox); filters.getChildren().add(filter); filter.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { int age; int index = ageFilterBox.getSelectionModel().getSelectedIndex(); age = (index == 0)? 0: (index == 1)? 21: (index == 2)? 31: 41; int salary; index = salaryFilterBox.getSelectionModel().getSelectedIndex(); salary = (index == 0)? 0 : (index == 1)? 151 : (index == 2)? 251:501; boolean relate; index = relationshipFilterBox.getSelectionModel().getSelectedIndex(); relate = (index == 1)? true: (index == 2)? false: true; List<User> list; if(index != 0) { list = users.stream(). filter(u -> u.age > age). filter(u -> u.salary > salary). filter(u -> u.married == relate). collect(Collectors.toList()); } else { list = users.stream(). filter(u -> u.age > age). filter(u -> u.salary > salary). collect(Collectors.toList()); } String res = ""; for(User u: list) { res += u.toString() + ", "; } resultFilter.setText(res); } }); } HBox filters = new HBox(); ComboBox<String> ageFilterBox = new ComboBox<>(); ComboBox<String> salaryFilterBox = new ComboBox<>(); ComboBox<String> relationshipFilterBox = new ComboBox<>(); Button filter = new Button("filter"); Text resultFilter = new Text(); }

Feito!
Código completoClasse da empresa (principal)
package company; import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.Insets; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.ComboBox; import javafx.scene.control.TextField; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.text.Text; import javafx.stage.Stage; public class Company extends Application { @Override public void start(Stage primaryStage) throws Exception { users.add(new User("Alice", 20, 150, false)); users.add(new User("Bob", 34, 300, true)); users.add(new User("Peter", 18, 100, false)); users.add(new User("Kate", 38, 300, true)); users.add(new User("Steve", 31, 250, true)); users.add(new User("Alan", 62, 500, true)); users.add(new User("Julia", 33, 320, true)); users.add(new User("Patric", 37, 300, true)); users.add(new User("Alexander", 34, 280, true)); users.add(new User("George", 28, 180, true)); users.add(new User("Mary", 22, 190, false)); userBox.getItems().addAll(users); ageFilterBox.getItems().addAll( "no matter", "over 20", "over 30", "over 40" ); salaryFilterBox.getItems().addAll( "no matter", "over 150", "over 250", "over 500" ); relationshipFilterBox.getItems().addAll( "no matter", "married", "single" ); root.getChildren().add(strings); strings.setPadding(new Insets(10, 30, 10, 30)); strings.setSpacing(20); strings.getChildren().add(new Text("Select the user")); strings.getChildren().add(buttonBox); strings.getChildren().add(new Text("Change the salary")); strings.getChildren().add(changeSalaryBox); strings.getChildren().add(new Text("Add new User")); strings.getChildren().add(addUserBox); strings.getChildren().add(filters); strings.getChildren().add(resultFilter); buttonBox.setSpacing(10); buttonBox.getChildren().add(userBox); buttonBox.getChildren().add(buttonGetInfo); buttonBox.getChildren().add(textInfo); changeSalaryBox.setSpacing(10); changeSalaryBox.getChildren().add(buttonChangeSalary); changeSalaryBox.getChildren().add(howMuchChange); addUserBox.setSpacing(10); addUserBox.getChildren().add(new Text("Name: ")); addUserBox.getChildren().add(name); addUserBox.getChildren().add(new Text("Age: ")); addUserBox.getChildren().add(age); addUserBox.getChildren().add(new Text("Salary: ")); addUserBox.getChildren().add(salary); addUserBox.getChildren().add(new Text("Married: ")); addUserBox.getChildren().add(married); addUserBox.getChildren().add(buttonAddUser); filters.setSpacing(10); filters.getChildren().add(new Text("Age")); filters.getChildren().add(ageFilterBox); filters.getChildren().add(new Text("Salary")); filters.getChildren().add(salaryFilterBox); filters.getChildren().add(new Text("Relationship")); filters.getChildren().add(relationshipFilterBox); filters.getChildren().add(filter); Scene scene = new Scene(root, WIDTH, HEIGHT); primaryStage.setTitle("Company"); primaryStage.setScene(scene); primaryStage.show(); buttonGetInfo.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { User u = (User) userBox.getSelectionModel().getSelectedItem(); if (u != null) { textInfo.setText("Age is " + u.getAge() + ", " + "Salary is " + u.getSalary() + ", " + "Relationship: " + u.getMarried()); } else { textInfo.setText("User not selected"); } } }); buttonChangeSalary.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { User u = (User) userBox.getSelectionModel().getSelectedItem(); if(u != null) { u.changeSalary(Integer.parseInt(howMuchChange.getText())); textInfo.setText("Age is " + u.getAge() + ", " + "Salary is " + u.getSalary() + ", " + "Relationshp: " + u.getMarried()); howMuchChange.clear(); } } }); buttonAddUser.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { String m = married.getText(); boolean mm = (m.equals("married"))? true:false; User u = new User(name.getText(), Integer.parseInt(age.getText()), Integer.parseInt(salary.getText()), mm); users.add(u); userBox.getItems().addAll(u); name.clear(); age.clear(); salary.clear(); married.clear(); } }); filter.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { int age; int index = ageFilterBox.getSelectionModel().getSelectedIndex(); age = (index == 0)? 0: (index == 1)? 21: (index == 2)? 31: 41; int salary; index = salaryFilterBox.getSelectionModel().getSelectedIndex(); salary = (index == 0)? 0 : (index == 1)? 151 : (index == 2)? 251:501; boolean relate; index = relationshipFilterBox.getSelectionModel().getSelectedIndex(); relate = (index == 1)? true: (index == 2)? false: true;
Classe de usuário
package company; public class User { User(String name, int age, int salary, boolean married) { this.name = name; this.age = age; this.salary = salary; this.married = married; } public void changeSalary(int x) { salary = (salary + x <= 0)? 0: salary + x; } public String toString() { return name; } public String getAge() { return String.valueOf(age); } public String getSalary() { return String.valueOf(salary); } public String getMarried() { return (married)? ("married"):("single"); } String name; int age; int salary; boolean married; }
O que foi isso
O programa em si não representa nenhuma utilidade e foi escrito
exclusivamente para se familiarizar com o JavaFX e suas ferramentas. Em alguns lugares, por exemplo, ao alterar um salário, as opções não são levadas em consideração quando o usuário não é selecionado ou quando uma linha é inserida: nesses casos, um erro será exibido. O mesmo se aplica aos campos em que as informações são inseridas ao adicionar novos funcionários. E, finalmente, ocorrerá um erro se algum valor não for selecionado em nenhuma das listas suspensas. Se desejar, todos podem adicionar seu próprio "desvio" desses momentos.
Ao escrever filtros, a propósito, usei o notório StreamAPI e
-expressões que encurtam o código acrescentam facilidade de entendimento e charme a ele.
Fontes:- JavaFX 8 - Centro de Ajuda do Oracle
- Um pouco sobre a criação e modificações históricas do JavaFX
- Artigo maravilhoso vedenin1980 sobre StreamAPI e -expressions