Boa tarde, querido leitor! Eu, um programador iniciante em Java, por um longo tempo não consegui me acostumar com a biblioteca Box2D. Em primeiro lugar, devido ao fato de ter sido escrito para C ++, e não haver documentação para isso, e eu não conheço a sintaxe C. Em segundo lugar, devido ao fato de que as lições detalhadas dessa biblioteca estão disponíveis apenas como uma extensão do libGDX. Depois de algumas semanas de luta, finalmente consegui entender como trabalhar com esta biblioteca e, neste artigo, falarei sobre isso (e mostrarei).
Eu trabalho no
Eclipse , o artigo será conectado a este ambiente de desenvolvimento. Para começar, faça o download do coletor
libGDX e faça uma compilação padrão. Precisamos de um aplicativo de desktop com a extensão Box2D. Em seguida, vá para o Eclipse, clique em Arquivo → Importar → Gradle → Projeto Gradle e especifique o caminho para sua montagem.
Aqui está uma foto mostrando a minha aparência. Adicionei o pacote Utils com a classe Constants à pasta Core, que contém apenas uma constante - o número de pixels por metro. Isso é para que o mundo não seja gigantesco.
Aqui está o código para a classe DesktopLauncher de com.mygdx.game.desktop:
Cole esse código na classe e esqueça-opackage com.mygdx.game.desktop; import com.badlogic.gdx.backends.lwjgl.LwjglApplication; import com.badlogic.gdx.backends.lwjgl.LwjglApplicationConfiguration; import com.mygdx.game.MyGdxGame; public class DesktopLauncher { public static void main(String[] arg) { LwjglApplicationConfiguration config = new LwjglApplicationConfiguration();
Haverá muito código, então eu vou envolvê-lo em spoilers. Somente a classe MyGdxGame do pacote com.mygdx.game é alterada.
Então, vamos tentar fazer uma coisa bastante simples em nosso aplicativo. O caminho da bola bate na parede das pranchas e elas se espalham. Algo assim:
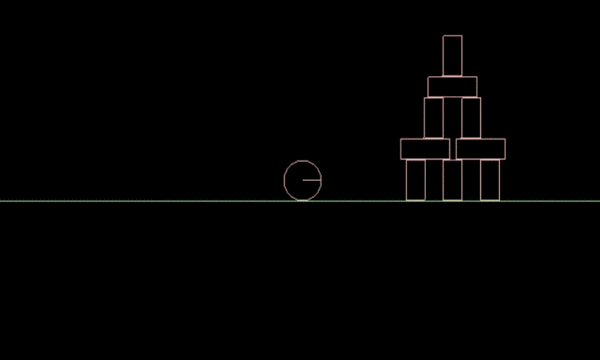
Ideia 1. Boliche package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.Input.Keys; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr;
Acabou ótimo, certo? Ao alterar vários parâmetros, você pode conseguir exatamente o que precisa! E não escreva tantas linhas de código.
Mas agora eu quero ver um golpe elástico. E que haja mais objetos no palco. Temos o seguinte resultado:
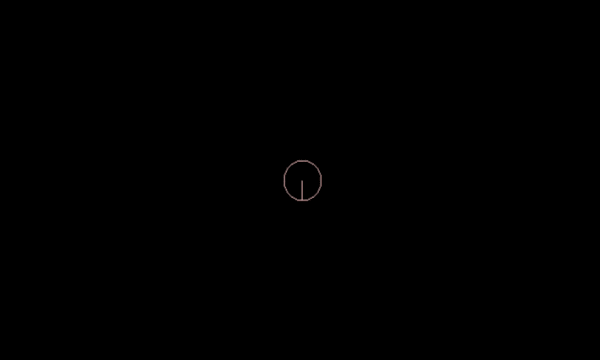
E aqui está o código: package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.physics.box2d.FixtureDef; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer();
Criar um carrinho no Box2D não é fácil. É necessário amarrar os corpos para que eles se movam como um todo. O GIF a seguir mostra apenas a essência.
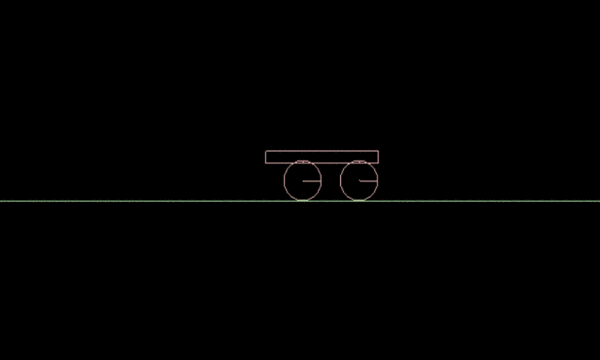
Como isso aconteceu? package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.Input; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body ball1; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer();
Se você olhar para o código, notará que este não é um carrinho de verdade, mas um pedaço de pau. Vá entusiastas! Há um
vídeo no Youtube onde um ICE de quatro tempos foi feito no Box2D. Somos piores? Esperando nos comentários pelo seu sucesso!
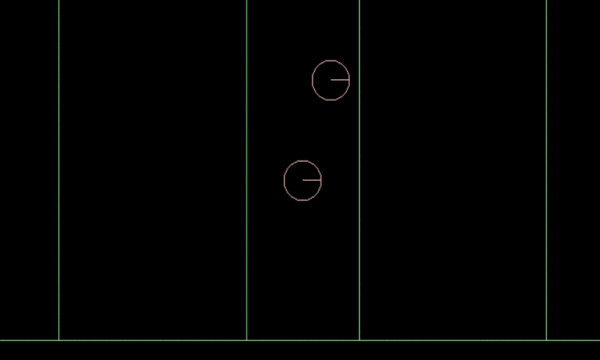
Mais confrontos! package com.mygdx.game; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.physics.box2d.Body; import com.badlogic.gdx.physics.box2d.BodyDef; import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer; import com.badlogic.gdx.physics.box2d.CircleShape; import com.badlogic.gdx.physics.box2d.FixtureDef; import com.badlogic.gdx.physics.box2d.PolygonShape; import com.badlogic.gdx.physics.box2d.World; import utils.Constants; public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body ball1; private Body ball2; private Body ball3; private Body ball4; private Body ball5; private Body floor; private Body wall; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer();
E o último GIF de hoje. O plano inclinado nos é familiar nas lições da física. Este código descreve como você pode obter uma forma mais complexa (teoricamente arbitrária), para que sejam abertas possibilidades ilimitadas para a física dos jogos.

Plano inclinado public class MyGdxGame extends ApplicationAdapter { SpriteBatch batch; Texture img; private OrthographicCamera camera; private boolean DEBUG = false; private World world; private Body ball; private Body floor; private Body wall; private Body plos; private Body verticals; private Body horizontals; private Box2DDebugRenderer b2dr; public void create() { float w = Gdx.graphics.getWidth(); float h = Gdx.graphics.getHeight(); camera = new OrthographicCamera(); camera.setToOrtho(false, w / 2, h / 2); world = new World(new Vector2(0, -9.8f), false); b2dr = new Box2DDebugRenderer(); ball = createPlayer(); floor = createfloor(); wall = createwall(430, 170); wall = createwall(-430, 170); plos = createplos(); } public void render() { update(Gdx.graphics.getDeltaTime()); Gdx.gl.glClearColor(0, 0, 0, 1); Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT); b2dr.render(world, camera.combined.scl(Constants.PPM)); } public void resize(int width, int height) { camera.setToOrtho(false, width / 2, height / 2); } public void dispose() { world.dispose(); b2dr.dispose(); } public void update(float delta) { world.step(1 / 60f, 6, 2); cameraUpdate(delta); } public void cameraUpdate(float delta) { Vector3 position = camera.position; position.x = ball.getPosition().x * Constants.PPM; position.y = ball.getPosition().y * Constants.PPM; camera.position.set(position); camera.update(); } public Body createPlayer() { Body pBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.DynamicBody; def.position.set(30 / Constants.PPM, 190 / Constants.PPM); def.fixedRotation = false; pBody = world.createBody(def); CircleShape shape = new CircleShape(); shape.setRadius(10 / Constants.PPM); pBody.createFixture(shape, 1.0f); def.bullet = true; shape.dispose(); return pBody; } public Body createfloor() { Body fBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.StaticBody; def.position.set(0, 0); def.fixedRotation = true; fBody = world.createBody(def); PolygonShape shape = new PolygonShape(); shape.setAsBox(480 / Constants.PPM, 70 / Constants.PPM); fBody.createFixture(shape, 0.001f); shape.dispose(); return fBody; } public Body createwall(int xo, int yo) { Body fBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.StaticBody; def.position.set(xo / Constants.PPM, yo / Constants.PPM); def.fixedRotation = true; fBody = world.createBody(def); PolygonShape shape = new PolygonShape(); shape.setAsBox(50 / Constants.PPM, 100 / Constants.PPM); fBody.createFixture(shape, 0.001f); shape.dispose(); return fBody; } public Body createplos() { Vector2[] vertices = new Vector2[3]; vertices[0] = new Vector2(0f , -0.6f ); vertices[1] = new Vector2(1f , -0.6f ); vertices[2] = new Vector2(1f , 1f); PolygonShape shape = new PolygonShape(); Body fBody; BodyDef def = new BodyDef(); def.type = BodyDef.BodyType.StaticBody; def.position.set(20 / Constants.PPM, 90 / Constants.PPM); def.fixedRotation = true; fBody = world.createBody(def); shape.set(vertices); fBody.createFixture(shape, 0.001f); shape.dispose(); return fBody; } }
Espero que, graças a este código, você seja capaz de entender o básico do Box2D e que aplicativos extremamente excelentes sejam criados! Obrigado por ler até o fim! Vou tentar responder a todas as perguntas nos comentários!