UPD:本教程已根据发行版本的发行版进行了更新。
好消息! mongoDB的官方go驱动程序已发布。
稍加思考,我决定从mongoDB官方网站翻译文章 ,而不是自己撰写材料(此翻译与文章不同)。
这是本教程中的内容:
- 安装mongo-go-driver
- 使用mongo-go-driver连接到mongoDB
- 使用BSON对象
- 使用CRUD方法
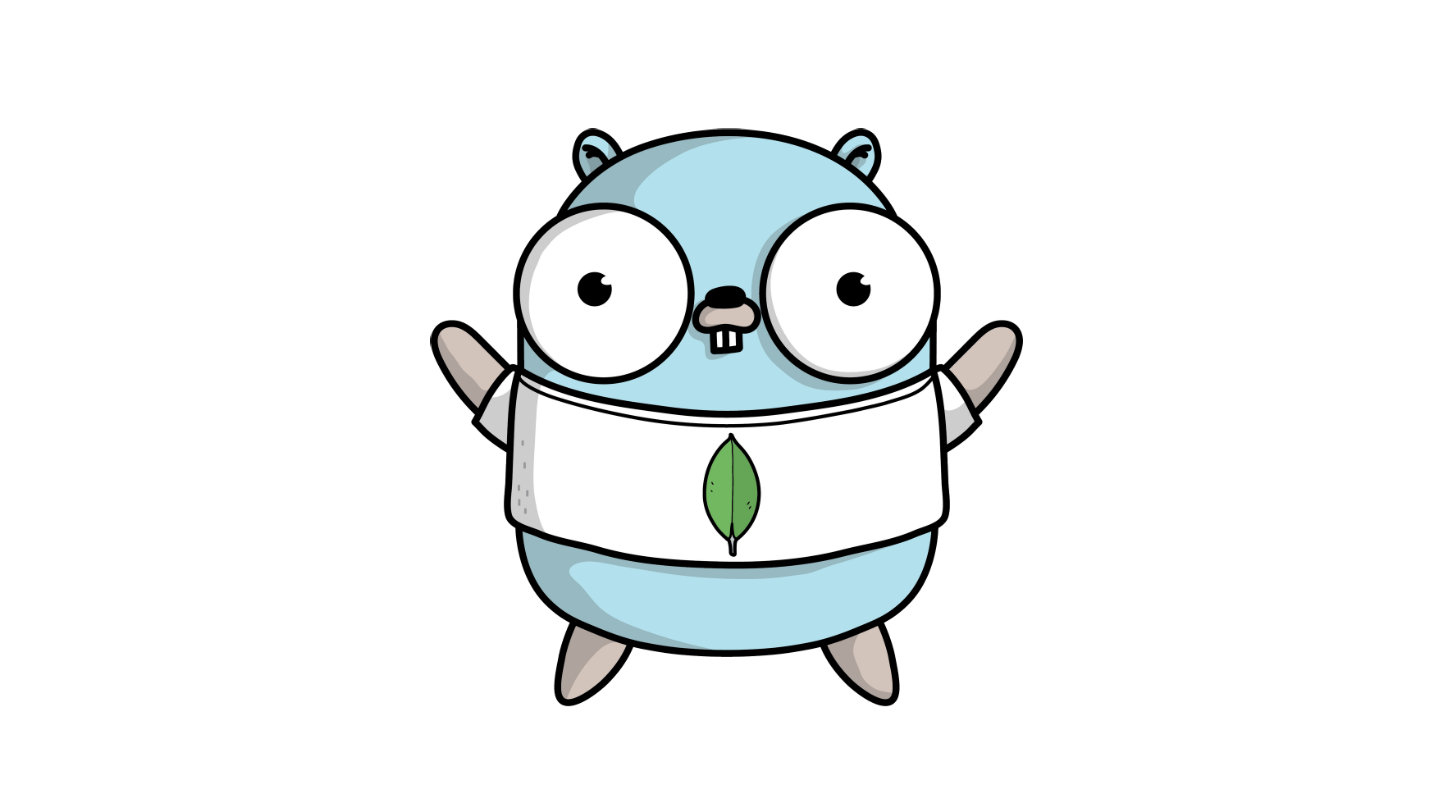
原始代码可以在官方文档中找到。
要使用本指南,您必须安装的MongoDB版本不低于2.6和Golang 1.10或更高版本。
安装mongo-go-driver
建议您使用dep安装驱动程序(当然,如果仍未切换到模块):
dep ensure -add "go.mongodb.org/mongo-driver/mongo@~1.0.0"
基金会创建
创建一个项目并添加具有以下内容的main.go文件:
package main import ( "context" "fmt" "log" "go.mongodb.org/mongo-driver/bson" "go.mongodb.org/mongo-driver/mongo" "go.mongodb.org/mongo-driver/mongo/options" "go.mongodb.org/mongo-driver/mongo/readpref" )
在此代码中,我们导入mongo-go-driver和一些标准库,并定义Trainer类型。
使用mongo-go-driver连接到mongoDB
导入mongo-go-driver后,我们就有机会使用mongo.NewClient()函数创建与mongoDB的连接。 我们有机会将上下文和包含与mongodb的连接地址的行作为参数传递给此函数。 如果需要,我们还可以将options.ClientOptions对象作为第三个参数传递,以微调驱动程序设置。
该文档详细介绍了可用的选项。
让我们将以下代码添加到主体函数主体中:
成功连接到mongoDB后,我们可以通过将以下代码添加到main函数的末尾,找到位于名为test的数据库中的trainers集合:
collection := client.Database("test").Collection("trainers")
建议您保持与mongoDB的连接处于打开状态,以便不必为每个请求打开和关闭连接。 但是,如果应用程序不再需要连接,则可以使用client.Disconnect()函数将其关闭,如下所示:
err = client.Disconnect(context.TODO()) if err != nil { log.Fatal(err) } fmt.Println("Connection to MongoDB closed.")
注释掉未使用的软件包。
运行我们的代码( go run main.go )以检查与mongoDB服务器的连接。
使用BSON对象
mongoDB中的JSON文档以称为BSON的二进制格式存储。 与其他以字符串和数字形式存储JSON数据的数据库不同,BSON编码添加了新类型,例如int , long , date , float和decimal128 。
这极大地简化了应用程序对数据的处理,分类和比较。 Go驱动程序具有两个用于表示BSON数据的类型族:D类型和RAW类型。
D系列包括四种类型:
- D :BSON文件。 此类型应在顺序很重要的情况下使用,例如MongoDB命令。
- M :无序字典(关联数组,映射)。 它与D相同,除了不保留顺序。
- A :BSON数组。
- E : D中的单个元素。
这是一个使用D类型构建的过滤器的示例,该过滤器搜索名称字段与Alice或Bob的值匹配的文档:
Raw类型系列用于检查字节切片。 您可以使用Lookup()从Raw类型提取单个元素。
当您在将BSON转换为另一种类型时需要摆脱额外的负担时,这将非常有用。
本教程仅使用D型系列。
使用CRUD方法
成功连接到数据库后,我们可以开始添加和修改集合中的数据。
集合类型包含允许您将查询发送到数据库的方法。
插入(创建)文件
首先,您需要创建一些新的Trainer结构以插入数据库:
ash := Trainer{"Ash", 10, "Pallet Town"} misty := Trainer{"Misty", 10, "Cerulean City"} brock := Trainer{"Brock", 15, "Pewter City"}
要嵌入单个文档,请使用collection.InsertOne()方法:
insertResult, err := collection.InsertOne(context.TODO(), ash) if err != nil { log.Fatal(err) } fmt.Println("Inserted a single document: ", insertResult.InsertedID)
要同时插入多个文档,存在collection.InsertMany()方法:
trainers := []interface{}{misty, brock} insertManyResult, err := collection.InsertMany(context.TODO(), trainers) if err != nil { log.Fatal(err) } fmt.Println("Inserted multiple documents: ", insertManyResult.InsertedIDs)
文件更新
collection.UpdateOne()方法允许您更新单个文档。 您需要创建一个过滤器来搜索数据库中的文档以及用于更新操作的文档。 您可以使用bson.D类型创建它们:
filter := bson.D{{"name", "Ash"}} update := bson.D{ {"$inc", bson.D{ {"age", 1}, }}, }
以下代码将找到一个文档,其中名称字段与Ash的值匹配,并将age的值增加1。
updateResult, err := collection.UpdateOne(context.TODO(), filter, update) if err != nil { log.Fatal(err) } fmt.Printf("Matched %v documents and updated %v documents.\n", updateResult.MatchedCount, updateResult.ModifiedCount)
文件搜寻
要查找文档,您将需要一个过滤器以及指向可以将结果解码为变量的指针。
要查找单个文档,请使用collection.FindOne() 。 此方法返回一个可以解码为变量的值。
我们将使用更新请求中使用的相同过滤器变量。
要查找多个文档,请使用collection.Find() 。
此方法返回Cursor 。 Cursor提供了一个文档流,您可以一次迭代和解码一个文档。
当Cursor中的文档用完时,应关闭Cursor 。 您还可以使用options软件包对Cursor进行微调。
在我们的示例中,我们对两个文档的发布设置了限制。
删除文件
您可以使用collection.DeleteOne()或collection.DeleteMany()删除文档。 我们将nil作为过滤器参数传递,它将匹配集合中的所有文档。 您还可以使用collection.Drop()删除整个集合。
filter := bson.M{} deleteResult, err := collection.DeleteMany(context.TODO(), filter) if err != nil { log.Fatal(err) } fmt.Printf("Deleted %v documents in the trainers collection\n", deleteResult.DeletedCount)
进一步的步骤
→本教程的最终代码在GitHub存储库中
→ GoDoc中提供了驱动程序文档
如有任何疑问,请通过google group mongo-go-driver与我们联系。
请将错误报告发送给MongoDB JIRA 。
我们很高兴收到您对Go Driver的反馈。