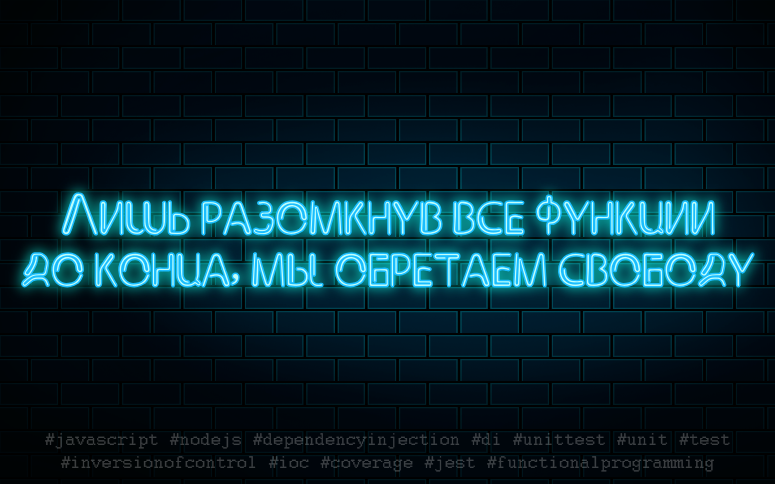
在本文中,我们将研究如何使用Dependency Injection编程模式以功能风格编写干净,易于测试的代码。 奖励是100%单元测试覆盖率。
本文中将使用的术语
本文的作者将牢记以下术语的这种解释,并理解这不是最终的真理,其他解释也是可能的。
- 依赖注入
这是一种编程模式,它假定函数和对象工厂的外部依赖关系是从外部以这些函数的参数形式出现的。 依赖注入是使用全局上下文中的依赖的替代方法。 - 净功能
这是一个函数,其结果仅取决于其参数。 此外,该功能不应有副作用。
我想立即保留一下我们正在考虑的功能没有副作用,但它们仍然可以具有通过依赖注入获得的功能。 因此,我们保留的功能纯洁。 - 单元测试
一个功能测试,检查该功能内的所有派生类是否与预期代码的作者完全相同。 在这种情况下,不是调用任何其他函数,而是使用对moks的调用。
我们在实践中了解
考虑一个例子。 有tick
的柜台工厂。 可以使用cancel
方法停止计数器。
const createCounter = ({ ticks, onTick }) => { const state = { currentTick: 1, timer: null, canceled: false } const cancel = () => { if (state.canceled) { throw new Error('"Counter" already canceled') } clearInterval(state.timer) } const onInterval = () => { onTick(state.currentTick++) if (state.currentTick > ticks) { cancel() } } state.timer = setInterval(onInterval, 200) const instance = { cancel } return instance } export default createCounter
我们看到了人类可读,可理解的代码。 但是有一个问题-不能在上面写普通的单元测试。 让我们看看有什么问题吗?
1)您无法访问cancel
, onInterval
内部的功能并分别对其进行测试。
2) onInterval
函数不能与cancel
函数分开测试,因为 第一个与第二个有直接链接。
3)使用外部依赖setInterval
, clearInterval
。
4)同样由于直接链接,无法将createCounter
函数与其他函数分开进行测试。
让我们解决问题1)2)-从闭包中删除cancel
, onInterval
,并通过pool
对象断开它们之间的直接链接。
export const cancel = pool => { if (pool.state.canceled) { throw new Error('"Counter" already canceled') } clearInterval(pool.state.timer) } export const onInterval = pool => { pool.config.onTick(pool.state.currentTick++) if (pool.state.currentTick > pool.config.ticks) { pool.cancel() } } const createCounter = config => { const pool = { config, state: { currentTick: 1, timer: null, canceled: false } } pool.cancel = cancel.bind(null, pool) pool.onInterval = onInterval.bind(null, pool) pool.state.timer = setInterval(pool.onInterval, 200) const instance = { cancel: pool.cancel } return instance } export default createCounter
我们解决问题3)。 我们在setInterval
和clearInterval
上使用了依赖注入模式,并将它们转移到pool
对象中。
export const cancel = pool => { const { clearInterval } = pool if (pool.state.canceled) { throw new Error('"Counter" already canceled') } clearInterval(pool.state.timer) } export const onInterval = pool => { pool.config.onTick(pool.state.currentTick++) if (pool.state.currentTick > pool.config.ticks) { pool.cancel() } } const createCounter = (dependencies, config) => { const pool = { ...dependencies, config, state: { currentTick: 1, timer: null, canceled: false } } pool.cancel = cancel.bind(null, pool) pool.onInterval = onInterval.bind(null, pool) const { setInterval } = pool pool.state.timer = setInterval(pool.onInterval, 200) const instance = { cancel: pool.cancel } return instance } export default createCounter.bind(null, { setInterval, clearInterval })
现在几乎一切都很好,但是仍然存在问题4)。 在最后一步,我们将依赖注入应用于每个函数,并通过pool
对象断开它们之间的其余连接。 同时,我们将一个大文件分成许多文件,以便以后编写单元测试变得更加容易。
结论
到底我们有什么? 一堆文件,每个文件包含一个清理功能。 代码的简单性和可理解性有所降低,但这远远超出了单元测试中100%覆盖率的补偿。

我还想指出,编写单元测试时,我们不需要对require
进行任何操作即可获取文件系统Node.js。
只有将所有功能放到最后,我们才能获得自由。