前言
我打招呼 最近,我面临在网站上设置推送通知的挑战。 这是我第一次遇到这个问题,
这篇文章对我有很大帮助。 它已经包含了服务器端的描述,但是,在研究此主题的过程中,我发现了一种更便捷的方法来实现Firebase库本身的功能。 实际上,我想向您介绍一下他,因为 我在互联网上找不到明确的解释。
本文对于Node.js,Python和Go的程序员也可能很有用,因为该库也支持这些语言。
直截了当
在本文中,我只会谈论服务器端。
(您可以使用同一篇文章配置客户端部分)
因此:
- 首先,您需要转到站点 ,注册并创建一个项目。
- 接下来,在左上角,单击齿轮,然后选择“项目设置”。
- 转到“服务帐户”选项卡,选择我们感兴趣的语言,单击“创建私钥”并下载生成的文件
此JSON文件包含Firebase库所需的配置。
现在让我们来照顾服务器为了方便起见,请在application.properties中声明下载文件的路径。
fcm.service-account-file = /path/to/file.json
在pom.xml中添加必要的依赖项
<dependency> <groupId>com.google.firebase</groupId> <artifactId>firebase-admin</artifactId> <version>6.7.0</version> </dependency>
创建一个返回JSON的bean:
@ConfigurationProperties(prefix = "fcm") @Component public class FcmSettings { private String serviceAccountFile; public String getServiceAccountFile() { return this.serviceAccountFile; } public void setServiceAccountFile(String serviceAccountFile) { this.serviceAccountFile = serviceAccountFile; } }
配置对象
@Getter @Setter public class PushNotifyConf { private String title; private String body; private String icon; private String click_action; private String ttlInSeconds; public PushNotifyConf() { } public PushNotifyConf(String title, String body, String icon, String click_action, String ttlInSeconds) { this.title = title; this.body = body; this.icon = icon; this.click_action = click_action; this.ttlInSeconds = ttlInSeconds; } }
栏位:
- 标题-目录
- 正文-通知文字
- 图标-链接到图片
- click_action-用户单击通知时将转到的链接(带有名称,例如服务中的示例)
您可以添加其中的几个,但并不是每个浏览器都会显示所有内容(以下是Chroma的示例)
- ttlInSeconds-通知有效时间
服务,这将是发送通知的全部逻辑:
@Service public class FcmClient { public FcmClient(FcmSettings settings) { Path p = Paths.get(settings.getServiceAccountFile()); try (InputStream serviceAccount = Files.newInputStream(p)) { FirebaseOptions options = new FirebaseOptions.Builder() .setCredentials(GoogleCredentials.fromStream(serviceAccount)) .build(); FirebaseApp.initializeApp(options); } catch (IOException e) { Logger.getLogger(FcmClient.class.getName()) .log(Level.SEVERE, null, e); } } public String sendByTopic(PushNotifyConf conf, String topic) throws InterruptedException, ExecutionException { Message message = Message.builder().setTopic(topic) .setWebpushConfig(WebpushConfig.builder() .putHeader("ttl", conf.getTtlInSeconds()) .setNotification(createBuilder(conf).build()) .build()) .build(); String response = FirebaseMessaging.getInstance() .sendAsync(message) .get(); return response; } public String sendPersonal(PushNotifyConf conf, String clientToken) throws ExecutionException, InterruptedException { Message message = Message.builder().setToken(clientToken) .setWebpushConfig(WebpushConfig.builder() .putHeader("ttl", conf.getTtlInSeconds()) .setNotification(createBuilder(conf).build()) .build()) .build(); String response = FirebaseMessaging.getInstance() .sendAsync(message) .get(); return response; } public void subscribeUsers(String topic, List<String> clientTokens) throws FirebaseMessagingException { for (String token : clientTokens) { TopicManagementResponse response = FirebaseMessaging.getInstance() .subscribeToTopic(Collections.singletonList(token), topic); } } private WebpushNotification.Builder createBuilder(PushNotifyConf conf){ WebpushNotification.Builder builder = WebpushNotification.builder(); builder.addAction(new WebpushNotification .Action(conf.getClick_action(), "")) .setImage(conf.getIcon()) .setTitle(conf.getTitle()) .setBody(conf.getBody()); return builder; } }
我:-Firebase,为什么会有这么多版本?
Firebase:-因为
- 构造函数用于使用我们的JSON文件初始化FirebaseApp。
- sendByTopic()方法将通知发送给订阅给定主题的用户。
- subscriptionUsers()方法订阅用户的主题(topic)(clientTokens)。
可以异步执行,为此使用.subscribeToTopicAsync()
- sendPersonal()方法实现了向用户发送个人通知(clientToken)
- createBuilder()方法创建一条消息
结果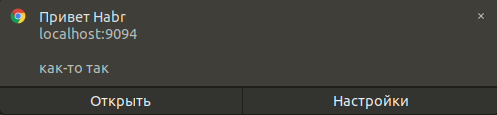
另一个浏览器

没有图标,因为Ubuntu :)
总结一下
本质上,Firebase库像这样为我们收集JSON:
{from: "Server key" notification: { title: " Habr" actions: (1) [ 0: Object { action: "https://habr.com/ru/top/", title: "" } ] length: 1 body: "- " image: "https://habrastorage.org/webt/7i/k5/77/7ik577fzskgywduy_2mfauq1gxs.png" } priority: "normal"}
在客户端,您已经可以根据需要对其进行解析了。
感谢您的关注!有用的链接:
firebase.google.com/docs/reference/admin/java/reference/com/google/firebase/messaging/WebpushNotificationhabr.com/zh-CN/post/321924/#otpravka-uvedomleniy-s-serverafirebase.google.com/docs/web/setup