十五或十五是一个在世界范围内流行的简单逻辑游戏的很好的例子。 为了解决这个难题,您需要按照从小到大的顺序依次排列数字。 这并不容易,但是很有趣。
在今天的教程中,我们展示了如何使用Eclipse在Java 8中开发15。 要开发UI,我们将使用Swing API。
我们提醒您: 对于所有“ Habr”读者来说,使用“ Habr”促销代码注册任何Skillbox课程时均可享受10,000卢布的折扣。
Skillbox建议:在线教育课程“专业Java开发人员” 。
游戏设计
在此阶段,您需要定义属性:
- 大小-比赛场地的大小;
- nbTiles-现场的点数。 nbTiles =尺寸*尺寸-1;
- Tiles是一个标记,它是一维整数数组。 每个标签将接收一个在[0,nbTiles]范围内的唯一值。 零表示一个空的正方形;
- blankPos-空正方形的位置。
游戏逻辑
您需要定义用于初始化新游戏位置的重置方法。 因此,我们为标签数组的每个元素设置值。 好吧,然后我们将blankPos放在数组的最后一个位置。
您还需要shuffle方法来随机播放标签数组。 我们在改组过程中不包含空标签,以使其保持原来的位置。
由于拼图的可能开始位置只有一半有解决方案,因此您需要检查混合结果,以确保通常可以解决当前布局。 为此,我们定义了isSolvable方法。
如果特定标签前面带有较高值的标签,则将其视为反转。 当空标签放置到位时,反转数必须为偶数才能解决。 因此,我们计算反转次数,如果次数为偶数,则返回true。
然后,定义isSolved方法很重要,以检查我们的15局对齐方式是否已解决。 首先,我们看一下空标记在哪里。 如果处于初始位置,则当前对齐方式是一个新的对齐方式,之前尚未确定。 然后,我们以相反的顺序遍历图块,如果标记值与相应的+1索引不同,则返回false。 否则,是时候在方法末尾返回true了,因为难题已经解决了。
另一种定义方法是newGame。 需要创建游戏的新实例。 为此,我们重置比赛场地,然后将其洗牌并继续直到可以解决比赛位置。
这是带有关键标签逻辑的示例代码:
private void newGame() { do { reset();
最后,您需要对阵列中斑点的移动进行编程。 稍后将通过回调调用此代码以响应光标移动。 我们的游戏将同时支持多个图块移动。 因此,在将屏幕上的按下位置转换为标签后,我们获得了空标签的位置,并寻找运动方向以同时支持其多个运动。
这是一个示例代码:
我们在Swing API上开发UI
现在该做界面了。 首先,我们参加Jpanel课程。 然后我们在场地上画点-为了计算每个点的大小,我们将使用游戏的构造函数参数中指定的数据:
gridSize = (dim - 2 * margin); tileSize = gridSize / size;
保证金也是游戏构造函数中指定的参数。
现在,您需要定义drawGrid方法以在屏幕上绘制网格和点。 我们分析标签的数组,并将坐标转换为用户界面的坐标。 然后在中心绘制带有相应编号的每个标签:
private void drawGrid(Graphics2D g) { for (int i = 0; i < tiles.length; i++) {
最后,我们重新定义paintComponent方法,该方法是JPane类的派生类。 然后,我们使用drawGrid方法,然后使用drawStartMessage方法显示一条消息,提示我们单击以开始游戏:
private void drawStartMessage(Graphics2D g) { if (gameOver) { g.setFont(getFont().deriveFont(Font.BOLD, 18)); g.setColor(FOREGROUND_COLOR); String s = "Click to start new game"; g.drawString(s, (getWidth() - g.getFontMetrics().stringWidth(s)) / 2, getHeight() - margin); } } private void drawCenteredString(Graphics2D g, String s, int x, int y) {
我们响应用户界面中的用户操作
为了使游戏顺利进行,有必要在UI中处理用户操作。 为此,请在Jpanel上添加MouseListener实现以及用于移动上面已经显示的位置的代码:
addMouseListener(new MouseAdapter() { @Override public void mousePressed(MouseEvent e) {
我们将代码放在GameOfFifteen类的构造函数中。 最后,我们调用newGame方法开始新游戏。
完整的游戏代码
在看到实际游戏之前,最后一步是将所有代码元素收集在一起。 结果如下:
import java.awt.BorderLayout; import java.awt.Color; import java.awt.Dimension; import java.awt.Font; import java.awt.FontMetrics; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.RenderingHints; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.util.Random; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.SwingUtilities;
最后,玩!
是时候开始游戏并进行实际检查了。 该字段应如下所示:
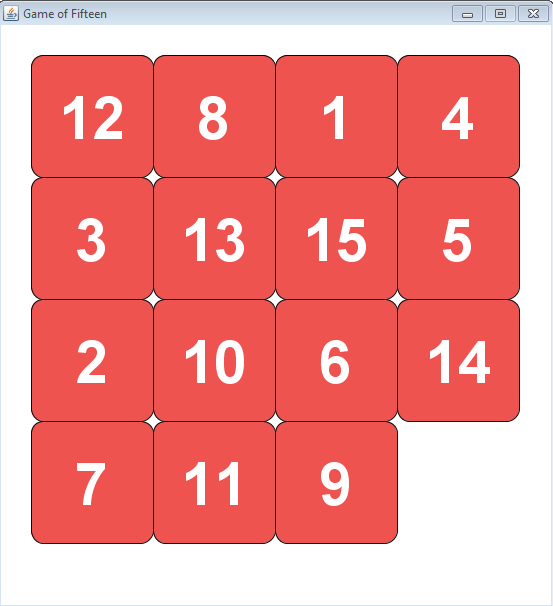
试图解决难题。 如果一切顺利,我们会得到:

仅此而已。 您还期望更多吗? :)
Skillbox建议: