我们将使用SFML库在C ++中将游戏制作为“标记”。 十五是一个著名的难题,看起来像这样:
在4x4的游戏环境中,随机放置15个骰子,每个骰子的数字从1到15,自由空间为1。 一次只能将一个骰子移动到一个空的位置。 游戏的目的是按照对应于其编号的顺序在游戏场上建立骰子。
因此,让我们开始吧。
启动Visual Studio并创建一个新的空项目。 您可以随意命名,我叫“ 15”。 在此项目中,创建一个新的main.cpp文件和一个空的main函数:
接下来,从
sfml-dev.org下载SFML库并解压缩。 解压缩的库包含我们需要的文件夹:
include ,
lib和
bin 。 在
其他包含目录的C / C ++部分的项目属性中,将路径添加到
包含文件夹:
在“
其他库目录”的“链接器”部分中
,将路径添加到
lib文件夹:
从
bin目录中,您需要复制DLL文件,并将其与我们项目的exe文件放置在目录中:
此外,在“链接器”部分的“输入”部分,您需要添加“
其他依赖项”中使用的库文件。 在我们的例子中,添加三个文件就足够了:sfml-system-d.lib,sfml-window-d.lib和sfml-graphics-d.lib:
文件名中的
-d符号表示它是调试版本,应在Debug配置中使用。 在发行版本的设置中,您将需要指定名称中没有
-d字符的文件。
在库
网站上有关于将SFML库连接到Visual Studio项目的很好的说明。
现在,让我们尝试在我们的项目中使用该库。 创建一个窗口并启动事件循环:
结果将是一个黑色背景为600 x 600像素的方形窗口:
可以使用鼠标或通过Esc键以通常的方式关闭窗口。 键盘按键处理程序也包含在消息处理循环中。
开始工作之前,我们需要某种字体在屏幕上显示文本。 例如,我采用了TrueType Calibri字体。
现在我们可以开始制作游戏了。
创建一个新的游戏类:
该班将负责游戏的操作和渲染比赛场地。 为此,我们将从SFML库的Drawable和Transformable类继承我们的类。
因此,我们开始描述我们的课程
Game.h #pragma once #include <SFML/Graphics.hpp> const int SIZE = 4; // const int ARRAY_SIZE = SIZE * SIZE; // const int FIELD_SIZE = 500; // const int CELL_SIZE = 120; // enum class Direction { Left = 0, Right = 1, Up = 2, Down = 3 }; class Game : public sf::Drawable, public sf::Transformable { protected: int elements[ARRAY_SIZE]; int empty_index; bool solved; sf::Font font; public: Game(); void Init(); bool Check(); void Move(Direction direction); public: virtual void draw(sf::RenderTarget& target, sf::RenderStates states) const; };
首先,我们连接图形库:
#include <SFML/Graphics.hpp>
在这里,我们声明了游戏所需的一些常量:
const int SIZE = 4;
我们还声明了我们的类型枚举,它确定了板的移动方向:
enum class Direction { Left = 0, Right = 1, Up = 2, Down = 3 };
最后,该类本身:
class Game : public sf::Drawable, public sf::Transformable { protected: int elements[ARRAY_SIZE]; int empty_index; bool solved; sf::Font font; public: Game(); void Init(); bool Check(); void Move(Direction direction); public: virtual void draw(sf::RenderTarget& target, sf::RenderStates states) const; };
我们拥有的最重要的东西是一个元素数组,其中包含与比赛场地的状态相对应的整数值。 数组中的元素从左到右,从上到下与比赛场地的元素相对应,也就是说,数组的前4个元素与场地的第一行相对应,后4个元素与第二行等等。
接下来,每个动作将要计算的两个变量是
Empty_index (数组中与空闲单元格相对应的索引)并已
求解 (表明难题已解决)。
此外,在类中设置了font变量,该变量确定在窗口中显示文本时将使用的字体。
现在,我们编写类方法的实现。
Game.cpp #include "Game.h" Game::Game() {
类构造函数从外部文件加载字体并调用游戏初始化方法:
Game::Game() {
游戏初始化方法以正确的顺序用元素填充数组,并设置已解决难题的符号:
void Game::Init() {
是的,最初,游戏将按照解决方案进行初始化,在游戏开始之前,我们将使用随机移动来混合骰子。
以下方法检查难题是否解决,并返回检查结果:
bool Game::Check() {
最后,一种实现游戏中牌移动的方法:
void Game::Move(Direction direction) {
类的最后一个方法是绘制比赛场地的方法:
画 void Game::draw(sf::RenderTarget& target, sf::RenderStates states) const { states.transform *= getTransform(); sf::Color color = sf::Color(200, 100, 200);
在渲染方法中,我们首先使用的是坐标变换,即乘以变换矩阵。 为了能够设置比赛场地的坐标,这是必需的。 接下来,使用SFML库的RectangleShape对象,绘制运动场的边界以及游戏中每个骰子的边界。 在骰子上,我们还绘制带有车牌号的文本。 另外,如果解决了难题,那么骰子的颜色也会不同。
现在该返回到主要功能了:
首先,加载字体并创建一个Text对象以显示带有键分配的文本行。 接下来,创建游戏对象并将字段的位置设置为具有坐标(50.50)的点-这就是我们从窗口边缘缩进的方式。
我决定通过键盘来控制游戏,因此每次按箭头键时,我们都会在游戏对象上调用Move方法,以沿相应的方向移动盘子。
按下F2键是新游戏的开始,因此在该事件的处理程序中,我们重新初始化游戏(这将导致骰子在其位置的放置),并将移动计数器的值设置为100。此计数器进一步用于在随机方向执行移动,直到不会重置,骰子也不会混合。 因此,我们肯定会获得解决难题的状态。
基本上就可以了,编译,组装,运行:
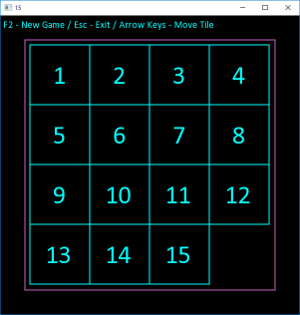

在本文中,我展示了如何使用SFML库快速创建一个简单的C ++游戏。 但是,程序本身的体系结构远非理想。 在下一篇文章中,我们将尝试对此做一些事情。