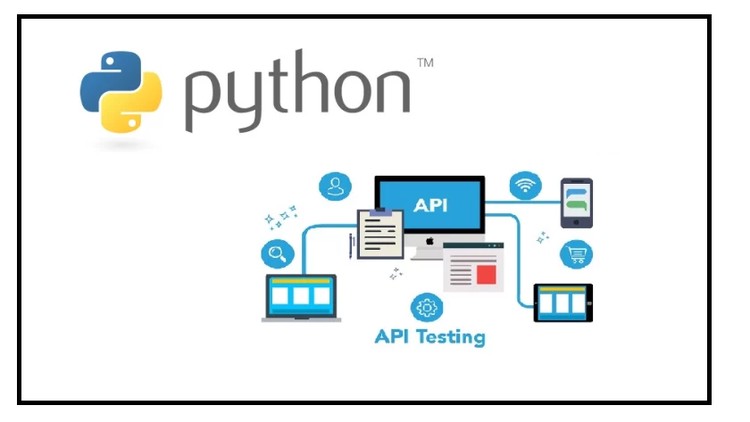
如果您正在阅读本文,您可能已经熟悉使用API(应用程序编程接口)打开的可能性。
通过向您的应用程序添加许多开放的API之一,您可以扩展此应用程序的功能或用必要的数据进行补充。 但是,如果您开发了想要与社区共享的独特功能,该怎么办?
答案很简单:您需要
创建自己的API 。
尽管起初看起来似乎是一项艰巨的任务,但实际上一切都很简单。 我们将向您展示如何使用Python执行此操作。
您需要开始什么
要开发API,您需要:
通过以下命令执行安装:
pip install flask-restful
推荐给初学者的免费密集编程:
C#中的Telegram机器人开发 -8月26日至28日。 免费密集型软件,可让您了解助手机器人的工作方式,使用Telegram API的功能以及其他细微差别。 最好的三个参加者将从Skillbox那里获得30,000卢布 。
开始之前我们将开发具有基本
CRUID功能的RESTful API。
为了完全理解该问题,让我们看一下上面提到的两个术语。
什么是REST?REST API(代表性状态传输)是一种使用HTTP请求交换数据的API。
REST API必须满足某些条件:
- 客户端-服务器体系结构:客户端与用户界面交互,服务器与后端和数据仓库交互。 客户端和服务器是独立的,它们中的任何一个都可以相互替换。
- 无状态-服务器上没有存储任何客户端数据。 会话状态存储在客户端。
- 可缓存性-客户端可以缓存服务器响应以提高整体性能。
什么是CRUD?CRUD是一种编程概念,描述了四个基本操作(创建,读取,更新和删除)。
在REST API中,请求类型和请求方法负责诸如发布,获取,放置,删除之类的操作。
现在我们已经弄清楚了基本术语,您可以开始创建API。
发展历程
让我们创建一个关于人工智能的报价存储库。 人工智能是当今发展最快的技术之一,Python是使用AI的流行工具。
借助此API,Python开发人员可以快速获取有关AI的信息并受到新开发的启发。 如果开发人员对此主题有宝贵的想法,则可以将其添加到存储库中。
让我们从导入必要的模块并配置Flask开始:
from flask import Flask from flask_restful import Api, Resource, reqparse import random app = Flask(__name__) api = Api(app)
在此片段中,Flask,Api和Resource是我们需要的类。
Reqparse是一个Flask-RESTful请求解析接口...您还将需要一个随机模块来显示随机报价。
现在,我们将创建一个AI报价存储库。
每个回购条目将包含:
由于这只是培训的示例,因此我们将所有条目保存在Python列表中。 在实际的应用程序中,我们很可能会改用数据库。
ai_quotes = [ { "id": 0, "author": "Kevin Kelly", "quote": "The business plans of the next 10,000 startups are easy to forecast: " + "Take X and add AI." }, { "id": 1, "author": "Stephen Hawking", "quote": "The development of full artificial intelligence could " + "spell the end of the human race… " + "It would take off on its own, and re-design " + "itself at an ever increasing rate. " + "Humans, who are limited by slow biological evolution, " + "couldn't compete, and would be superseded." }, { "id": 2, "author": "Claude Shannon", "quote": "I visualize a time when we will be to robots what " + "dogs are to humans, " + "and I'm rooting for the machines." }, { "id": 3, "author": "Elon Musk", "quote": "The pace of progress in artificial intelligence " + "(I'm not referring to narrow AI) " + "is incredibly fast. Unless you have direct " + "exposure to groups like Deepmind, " + "you have no idea how fast — it is growing " + "at a pace close to exponential. " + "The risk of something seriously dangerous " + "happening is in the five-year timeframe." + "10 years at most." }, { "id": 4, "author": "Geoffrey Hinton", "quote": "I have always been convinced that the only way " + "to get artificial intelligence to work " + "is to do the computation in a way similar to the human brain. " + "That is the goal I have been pursuing. We are making progress, " + "though we still have lots to learn about " + "how the brain actually works." }, { "id": 5, "author": "Pedro Domingos", "quote": "People worry that computers will " + "get too smart and take over the world, " + "but the real problem is that they're too stupid " + "and they've already taken over the world." }, { "id": 6, "author": "Alan Turing", "quote": "It seems probable that once the machine thinking " + "method had started, it would not take long " + "to outstrip our feeble powers… " + "They would be able to converse " + "with each other to sharpen their wits. " + "At some stage therefore, we should " + "have to expect the machines to take control." }, { "id": 7, "author": "Ray Kurzweil", "quote": "Artificial intelligence will reach " + "human levels by around 2029. " + "Follow that out further to, say, 2045, " + "we will have multiplied the intelligence, " + "the human biological machine intelligence " + "of our civilization a billion-fold." }, { "id": 8, "author": "Sebastian Thrun", "quote": "Nobody phrases it this way, but I think " + "that artificial intelligence " + "is almost a humanities discipline. It's really an attempt " + "to understand human intelligence and human cognition." }, { "id": 9, "author": "Andrew Ng", "quote": "We're making this analogy that AI is the new electricity." + "Electricity transformed industries: agriculture, " + "transportation, communication, manufacturing." } ]
现在,我们需要创建一个资源类Quote,这将确定我们API端点的操作。 在类中必须声明四个方法:get,post,put,delete。
让我们从GET方法开始。通过指定其ID可以获取特定的报价,如果未指定ID,则可以使用随机报价。
class Quote(Resource): def get(self, id=0): if id == 0: return random.choice(ai_quotes), 200 for quote in ai_quotes: if(quote["id"] == id): return quote, 200 return "Quote not found", 404
如果ID包含默认值,即GET方法,则GET方法返回随机报价。 调用该方法时,未设置ID。
如果已指定,则该方法将在引号中搜索并找到包含指定ID的引号。 如果未找到任何内容,则显示消息“找不到报价,404”。
请记住:如果请求成功,则该方法返回的HTTP状态为200,如果未找到该记录,则返回404。
现在让我们创建一个POST方法,以向存储库添加新的报价
键入时,它将收到每个新报价的标识符。 另外,POST将使用reqparse解析将在请求正文中使用的参数(作者和引文)。
def post(self, id): parser = reqparse.RequestParser() parser.add_argument("author") parser.add_argument("quote") params = parser.parse_args() for quote in ai_quotes: if(id == quote["id"]): return f"Quote with id {id} already exists", 400 quote = { "id": int(id), "author": params["author"], "quote": params["quote"] } ai_quotes.append(quote) return quote, 201
在上面的代码中,POST方法接受了报价ID。 然后,使用reqparse,他从查询中获得了作者和报价,并将它们存储在params字典中。
如果已经存在具有指定ID的报价,则该方法将显示相应的消息和代码400。
如果尚未创建具有指定ID的报价,则该方法将创建具有指定ID和作者以及其他参数的新记录。 然后,他将一个条目添加到ai_quotes列表中,并返回一个带有新引号的条目以及代码201。
现在创建一个PUT方法来修改存储库中的现有报价。
def put(self, id): parser = reqparse.RequestParser() parser.add_argument("author") parser.add_argument("quote") params = parser.parse_args() for quote in ai_quotes: if(id == quote["id"]): quote["author"] = params["author"] quote["quote"] = params["quote"] return quote, 200 quote = { "id": id, "author": params["author"], "quote": params["quote"] } ai_quotes.append(quote) return quote, 201
与前面的示例类似,PUT方法采用ID和输入并使用reqparse解析报价参数。
如果存在具有指定ID的引用,则该方法将使用新参数对其进行更新,然后显示带有代码200的更新的引用。如果没有具有指定ID的引用,则将使用代码201创建新记录。
最后,让我们创建一个DELETE方法来删除不再鼓舞人心的报价。
def delete(self, id): global ai_quotes ai_quotes = [qoute for qoute in ai_quotes if qoute["id"] != id] return f"Quote with id {id} is deleted.", 200
此方法在您键入时获取报价ID,并使用通用列表更新ai_quotes列表。
现在我们已经创建了所有方法,我们所要做的就是将资源添加到API,设置路径并运行Flask。
api.add_resource(Quote, "/ai-quotes", "/ai-quotes/", "/ai-quotes/<int:id>") if __name__ == '__main__': app.run(debug=True)
我们的REST API服务已准备就绪!
接下来,我们可以使用以下命令在控制台中运行代码,以将代码保存在app.py文件中:
python3 app.py
如果一切顺利,那么我们将得到以下内容:
*调试模式:打开
*运行于127.0.0.1:5000 /(按CTRL + C退出)
*用统计重启
*调试器处于活动状态!
*调试器PIN:XXXXXXX测试API创建API后,需要对其进行测试。
可以使用curl控制台实用程序或Insomnia REST客户端或通过将API发布到Rapid API来完成此操作。
发布我们的APIRapidAPI是世界上最大的市场,拥有10,000多个API(以及大约100万开发人员)。
RapidAPI不仅提供用于与第三方API一起使用的单一接口,而且还使您能够快速轻松地发布自己的API。
为此 ,您首先需要将其发布在网络上的某些服务器上。 在我们的例子中,我们将使用
Heroku 。 与他一起工作不会造成任何困难(
您可以在此处了解有关他的更多信息 )。
如何在Heroku上发布API1.安装Heroku。第一步是注册并安装Heroku命令行界面(CLI)。 这适用于Ubuntu 16+。
sudo snap install heroku --classic然后登录:
heroku登录2.添加必要的文件。现在,我们需要将要发布的文件添加到应用程序的文件夹中:
- requirements.txt以及所需的Python模块列表;
- Procfile,指示必须执行哪些命令才能启动应用程序;
- .gitignore-排除服务器上不需要的文件。
requirements.txt文件将包含以下几行:
请注意:由于我们需要在服务器上运行应用程序,因此我们在列表中添加了gunicorn(Python WSGI HTTP Server)。
Procfile将包含:
网站:gunicorn app:app.gitignore的内容:
*.pyc __pycache__/
现在已经创建了文件,让我们初始化git repo并提交:
git init git add git commit -m "First API commit"
3.创建一个新的Heroku应用程序。 heroku create
我们将master分支发送到远程Heroku存储库:
git push heroku master
现在,您可以使用以下命令打开API服务:
heroku ps:scale web=1 heroku open
该API将在
your-random-heroku-name.herokuapp.com/ai-quotes上提供 。
如何将Python API添加到RapidAPI市场在Heroku上发布API服务后,您可以将其添加到Rapid API。 这是有关此主题的
详细文档 。
1.创建一个RapidAPI帐户。
注册一个免费帐户-可以使用Facebook,Google,GitHub来完成。
2.将API添加到控制面板。
3.接下来,输入有关您的API的常规信息。
4.单击“添加API”后,将出现一个新页面,您可以在其中输入有关我们API的信息。
5.现在,您可以手动输入API端点,也可以使用OpenAPI下载swagger文件 。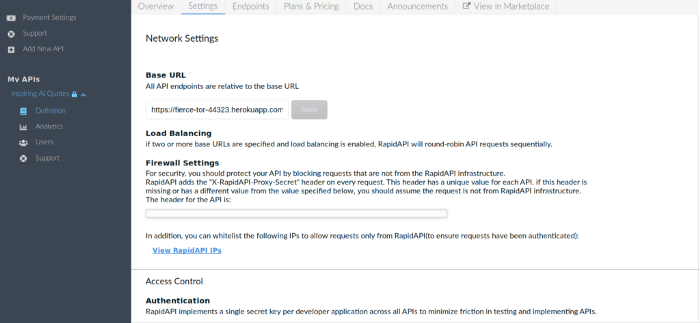
好了,现在我们需要在“端点”页面上设置API的端点。 在我们的例子中,端点对应于CRUD概念(获取,发布,放置,删除)。
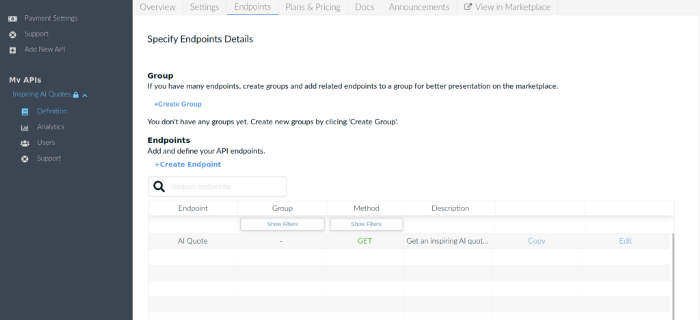
接下来,您需要创建GET AI Quote端点,该端点显示一个随机引号(如果ID为默认值)或指定ID的引号。
要创建端点,请单击“创建端点”按钮。

对所有其他API端点重复此过程。 仅此而已! 恭喜,您已经发布了API!
如果一切顺利,API页面将如下所示:

结论
在本文中,我们探讨了在Python中创建自己的RESTful API服务的过程,以及将API发布到Heroku云并将其添加到RapidAPI目录的过程。
但是在测试版本中,仅显示了API开发的基本原理-未考虑安全性,容错性和可伸缩性等细微差别。
在开发真实的API时,必须考虑所有这些因素。