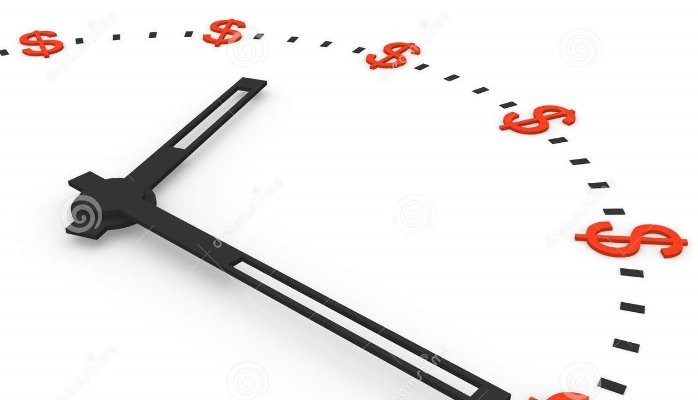
该出版物介绍了内置工具的软件实现,这些工具用于收集和累积用C / C ++ / C#编写的应用程序运行时的度量标准信息。
所描述方法的实质是基于在应用程序的程序代码中包含“控制点”,用于提取有关结构组件(方法,函数和{}块)执行时间的数据。 提取的度量标准信息将存储在内部数据库中,其内容在应用程序末尾转换为保存在文件中的文本报告形式。 使用内置控制执行时间的方法的适当性是由于需要识别代码的问题区域,分析应用程序暂时降级的原因:全部或部分,或表现在某些源数据集上。
给定的C ++ / C#源代码示例演示了所描述方法的可能实现。
引言
在软件开发的每次迭代(例如,下一版本的发行)中进行软件应用程序的开发包括以下基本步骤:
- 功能开发和测试;
- 优化RAM的消耗资源;
- 运行时指标的稳定化。
这些步骤需要大量的开发人员,不仅需要创造性的工作(例如有效算法的开发和实现,构建灵活的软件体系结构等),而且还需要日常工作。 后一类包括旨在稳定应用程序执行时间指标的活动。 在许多情况下,当开发人员面临性能下降时,这是一个相当痛苦的过程,这是扩展软件产品的功能,重建软件体系结构以及应用程序中出现新线程的结果。 同时,退化源需要付出一定的努力来检测它们,这不仅通过开发人员的高度勤奋和责任感(必要条件),而且还通过用于这些目的的工具组成(充分条件)来实现。
解决应用程序时间指标问题的一种有效方法是使用专用软件产品,例如GNU
gprof 。 通过此类工具生成的报告分析,您可以识别“瓶颈”(类方法和函数),这些瓶颈占执行整个应用程序所花费的大量时间。 同时,在方法和过程的执行上花费的时间的有效性肯定由开发人员确定。
还应注意,该类的软件产品通常在类和函数的方法级别上对程序代码的执行时间进行度量分析,而忽略以下级别(但从问题分析的角度来看仍然很重要):
{...}同时,直到try-catch块
一直执行到执行,否则在其中发生不少于执行时间的开销。
接下来,考虑用于执行执行时间工具的内置控制的一种可能解决方案的主要内容,该工具旨在提取和积累有关受控软件块的时间度量的详细信息,并为开发人员随后生成报告。
检索运行时数据的方法
任何软件应用程序的功能都可以解释为
抽象机器,它具有一组有限的唯一
状态 {St},并在它们之间具有过渡
{Tr} 。
在这种方法的框架中,应将应用程序中的任何执行流程都解释为其状态及其之间的转换的有序序列。 在这种情况下,执行时间成本的估算是通过将整个通过状态集上的时间度量求和而忽略从一个状态到另一状态的转换成本(可忽略的值)来执行的。
通过指定的控制点在应用程序执行时提取和累积数据是下面描述的内置控制工具解决的主要任务。
对于源代码中声明的每个断点,请放置
PROFILE_ENTRY C ++宏,记录其在应用程序执行期间的通过次数,以及时间度量-从检查点传递到程序层次结构的下一级(包括块,类方法,函数等)起,应用程序处于状态的总时间。如下图所示。
控制点的控制(初始注册和时间度量的计算)由在单个实例中创建的
“ timeManager”对象执行。 每次通过控制点的事件都由对象
'timeManager'记录
,并在第一次通过时将其记录为可观察到的
'registerEntry' 。
在每次通过控制点时,
都会创建一个
timerObject对象,以固定其创建时间。 当应用程序从软件层次结构的当前级别退出时,执行时间固定在检查点。 这时,对象的timerObject会自动销毁,并伴随其“生存期” T的计算。结果,
timeManager增加了检查点通过的次数以及在其中花费的时间
。 对于所有设置的控制点,当应用程序终止时,
timeManager会在报告的后续版本中累积数据。
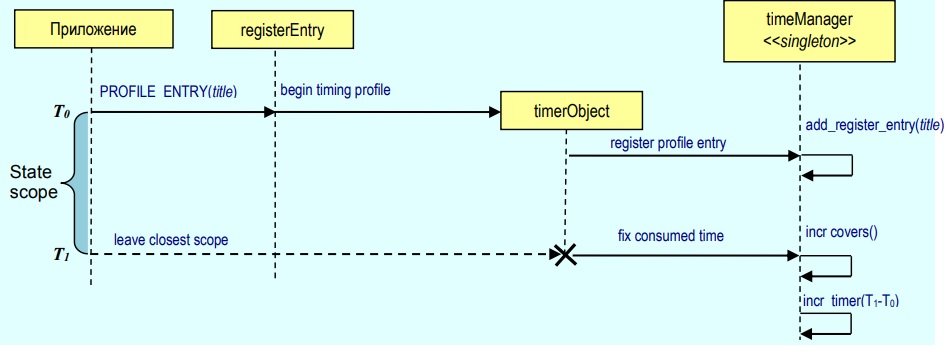
以下是源C ++代码,该代码实现了用于控制应用程序执行时间的内置工具。
演示应用程序的结构如下所示,以使用内置的运行时控制工具为例,并获得了结果表(有关详细信息,请参见
附录1。演示应用程序的源代码 )。
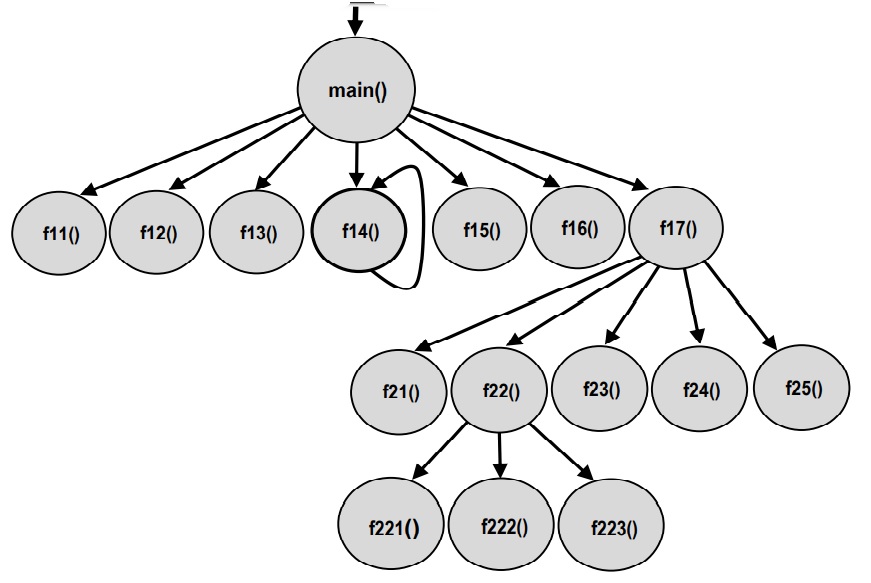
附录2。C#应用程序执行时间的内置控件的方法的源代码提供了C#中内置控件的方法的类似实现。
作者在分析费力(从计算角度出发)的方法和过程的执行时间时使用了
TimeWatcher.StartWatch()和
TimeWatcher.StopWatch()对,这是
EREMEX开发的
Delta Design软件产品的一部分,该产品是电子设备的计算机辅助设计系统。
以下是有关上述产品功能之一的时间指标的简短报告示例。
简要结论
所描述的工具可用于在其程序代码的各个部分中收集有关应用程序执行时间的数据,尤其是它们允许:
- 根据应用程序中执行线程的时间指标收集和累积数据;
- 对程序代码的执行时间进行估计以符合基本语言结构;
- 通过打开和关闭应用程序执行流的相应部分上的内置控制工具来管理提取的数据量;
- 开发并应用回归测试以监视应用程序时间指标的稳定性(并检测退化)。
总之,应该指出的是,在本出版物的范围之外,还有在
多线程应用程序中使用所描述的内置控制工具的问题,并且没有以任何形式对通过时间度量获得的数据的准确性进行分析。 后者是由于以下事实:在实践中,当确定应用程序临时降级的原因时,与应用程序
的软件组件之间的执行时间成本 的相对分布 有关的数据主要相关
。 在这方面,关于获得的数据的准确性的问题逐渐淡出背景。
附录1.演示应用程序的源代码
INITIALIZE_PROFILER int main(int argc, char * argv[]) {
补充2.内置运行时控件C#应用程序的源代码