5月下旬,Microsoft发布了Xamarin.Forms Shell版本-该外壳程序旨在简化跨平台移动应用程序的创建,并包括以下功能:侧菜单,选项卡,导航,搜索。
让我们首先在Visual Studio 2019中创建一个空的Xamarin.Forms项目。请注意,目前,Shell正式仅支持两个平台:iOS和Android,UWP仍在开发中。 我建议立即更新解决方案中的所有nuget软件包。

接下来,我们将创建从Shell派生的AppShell类,为此,我们将XAML文件添加到具有以下内容的常规项目中:
AppShell.xaml<?xml version="1.0" encoding="utf-8" ?> <Shell xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" x:Class="HelloShell.AppShell"> </Shell>
AppShell.xaml.cs namespace HelloShell { [XamlCompilation(XamlCompilationOptions.Compile)] public partial class AppShell : Shell { public AppShell() { InitializeComponent(); } } }
然后在文件
App.xaml.cs中,我们指示AppShell将充当MainPage:
public App() { InitializeComponent();
以及两个ContentPage页面:Page1和Page2。 同样,图像将在我们的测试应用程序中使用,因此我们会将它们添加到平台相关项目中,对于Resources => drawable文件夹中的android,对于Resources文件夹中的ios。
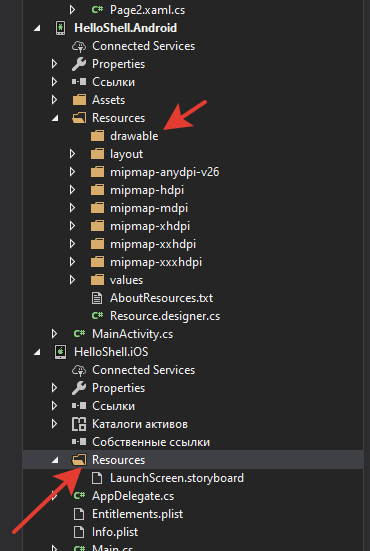
侧面菜单
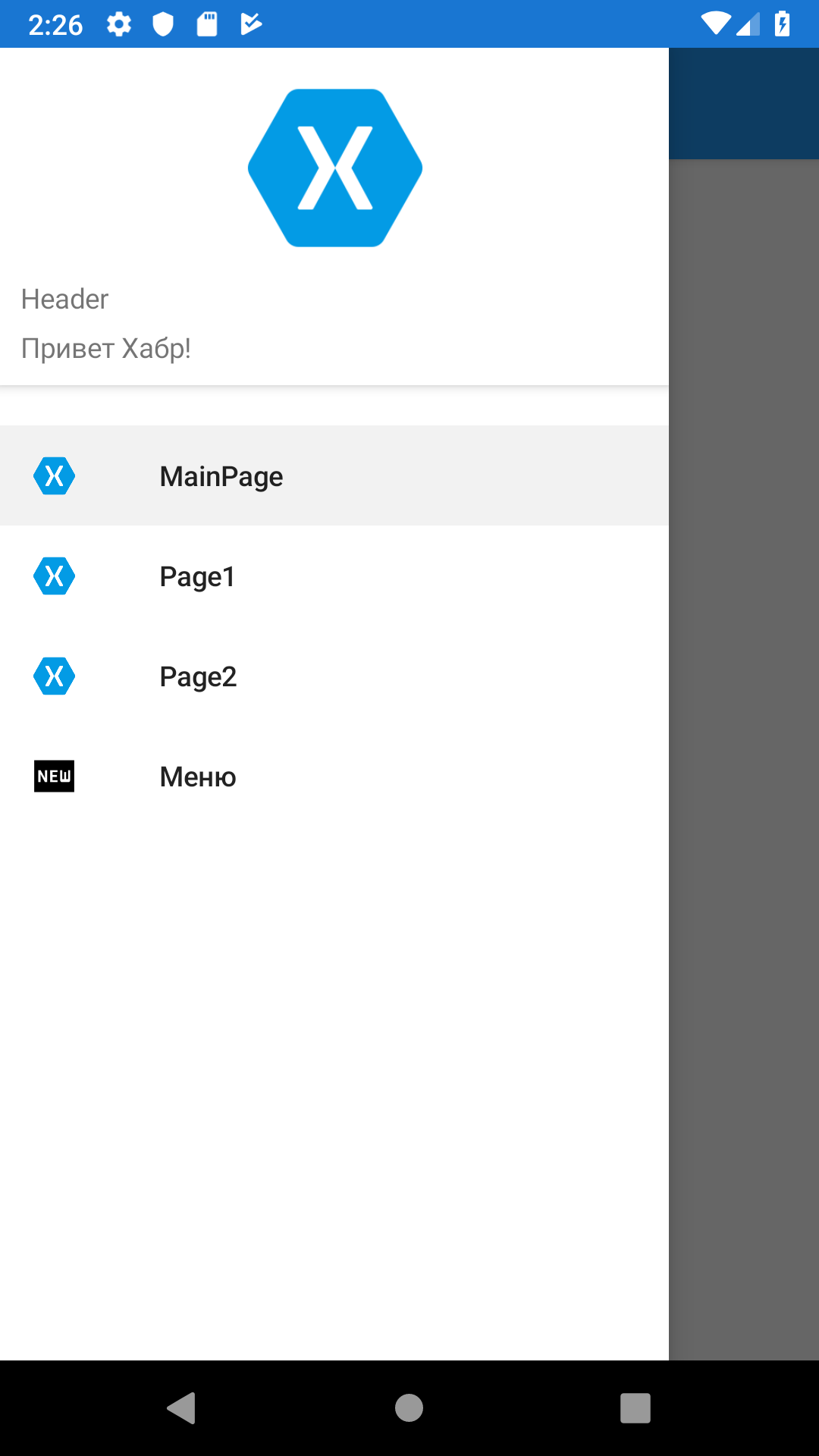
侧面菜单(通常称为汉堡菜单)是一种弹出菜单,可以通过按按钮或用特殊手势来调用,包括标题(Header),页面列表(弹出项目)和菜单(弹出菜单)
AppShell.xaml <?xml version="1.0" encoding="utf-8" ?> <Shell xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" xmlns:pages="clr-namespace:HelloShell" x:Class="HelloShell.AppShell"> <Shell.FlyoutHeader> <StackLayout BackgroundColor="White" Padding="10"> <Image HeightRequest="100" Source="xamarin.png" /> <Label Text="Header" /> <Label Text=" !"/> </StackLayout> </Shell.FlyoutHeader> <FlyoutItem Title="MainPage" Icon="xamarin.png"> <ShellContent ContentTemplate="{DataTemplate pages:MainPage}"/> </FlyoutItem> <FlyoutItem Title="Page1" Icon="xamarin.png"> <ShellContent ContentTemplate="{DataTemplate pages:Page1}"/> </FlyoutItem> <FlyoutItem Title="Page2" Icon="xamarin.png"> <ShellContent ContentTemplate="{DataTemplate pages:Page2}"/> </FlyoutItem> <MenuItem Clicked="MenuItem_Clicked" Text="" IconImageSource="item.png" /> </Shell>
AppShell.xaml.cs namespace HelloShell { [XamlCompilation(XamlCompilationOptions.Compile)] public partial class AppShell : Shell { public AppShell() { InitializeComponent(); } private async void MenuItem_Clicked(object sender, System.EventArgs e) { await DisplayAlert(""," !","OK"); } } }
标签

Xamarin.Forms Shell作为根模板可以支持上下选项卡及其组合:
AppShell.xaml.cs <?xml version="1.0" encoding="utf-8" ?> <Shell xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" xmlns:pages="clr-namespace:HelloShell" x:Class="HelloShell.AppShell"> <TabBar> <Tab Title="MainPage" Icon="xamarin.png"> <ShellContent ContentTemplate="{DataTemplate pages:MainPage}" /> </Tab> <Tab Title="Page1" Icon="xamarin.png"> <ShellContent Title="Main Page" Icon="xamarin.png" ContentTemplate="{DataTemplate pages:MainPage}" /> <ShellContent Title="Page2" Icon="xamarin.png" ContentTemplate="{DataTemplate pages:Page2}" /> </Tab> <Tab IsEnabled="False" Title="Page2" Icon="xamarin.png"> <ShellContent ContentTemplate="{DataTemplate pages:Page2}" /> </Tab> </TabBar> </Shell>
如您所见,这非常简单。 另一个优点是页面的有效加载,这使您仅在用户进入页面时才可以初始化页面,从而大大加快了应用程序的启动速度。
导览
Xamarin.Forms提供了改进的基于URI的导航,使您无需遵循严格的层次结构即可转到应用程序中的任何页面,而无需浏览导航堆栈中的所有页面即可返回。 为了使导航正常运行,需要注册页面,您可以使用Route属性在FlyoutItem,Tab和ShellContent的XAML标记中进行此操作
<Shell ...> <FlyoutItem ... Route="page1"> <Tab ... Route="page2"> <ShellContent ... Route="mainpage" /> <ShellContent ... Route="page3" /> </Tab> <ShellContent ... Route="page4" /> <ShellContent ... Route="page5" /> </FlyoutItem> <ShellContent ... Route="about" /> ... </Shell>
或用代码
Routing.RegisterRoute("page1", typeof(Page1));
使用以下命令进行导航
await Shell.Current.GoToAsync("//page2");
例如,我们对以下文件进行更改:
AppShell.xaml <TabBar> <Tab Route="main" Title="MainPage" Icon="xamarin.png"> <ShellContent ContentTemplate="{DataTemplate pages:MainPage}" /> </Tab> <Tab Title="Page1" Icon="xamarin.png"> <ShellContent Title="Main Page" Icon="xamarin.png" ContentTemplate="{DataTemplate pages:MainPage}" /> <ShellContent Route="page2" Title="Page2" Icon="xamarin.png" ContentTemplate="{DataTemplate pages:Page2}" /> </Tab> <Tab IsEnabled="False" Title="Page2" Icon="xamarin.png"> <ShellContent ContentTemplate="{DataTemplate pages:Page2}" /> </Tab> </TabBar>
MainPage.xaml <StackLayout VerticalOptions="CenterAndExpand"> <Button Text="Page2" Clicked="ToPage2" /> </StackLayout>
MainPage.xaml.cs public partial class MainPage : ContentPage { public MainPage() { InitializeComponent(); } private async void ToPage2(object sender, EventArgs e) { await Shell.Current.GoToAsync("//page2"); } }
Page2.xaml <ContentPage.Content> <StackLayout VerticalOptions="CenterAndExpand"> <Button Text="" Clicked="Back" /> </StackLayout> </ContentPage.Content>
Page2.xaml.cs public partial class Page2 : ContentPage { public Page2() { InitializeComponent(); } private async void Back(object sender, EventArgs e) { await Shell.Current.GoToAsync("//main"); } }
搜寻
Xamarin.Forms Shell具有SearchHandler类提供的内置搜索功能。 为了向页面添加搜索功能,我们将创建一个派生自SearchHandler的PetSearchHandler类,并重写OnQueryChanged和OnItemSelected方法。 当用户在搜索字段中输入文本并采用两个参数:oldValue和newValue时,将触发OnQueryChanged方法,它们分别包含先前的搜索查询和新的搜索查询。
当用户选择搜索结果并将对象(在这种情况下为Animal)作为参数时,将执行SelectedItem方法。
例如,创建动物模型
模型/ Animal.cs public class Animal { public string Name { get; set; } public string ImageUrl { get; set; } }
PetData类,它将包含我们最喜欢的猫和狗的集合
数据/ PetData.cs public static class PetData { public static IList<Animal> Pets { get; private set; } static PetData() { Pets = new List<Animal>(); Pets.Add(new Animal { Name = "Afghan Hound", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/6/69/Afghane.jpg" }); Pets.Add(new Animal { Name = "Alpine Dachsbracke", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/thumb/2/23/Alpejski_gończy_krótkonożny_g99.jpg/320px-Alpejski_gończy_krótkonożny_g99.jpg" }); Pets.Add(new Animal { Name = "American Bulldog", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/5/5e/American_Bulldog_600.jpg" }); Pets.Add(new Animal { Name = "Abyssinian", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/thumb/9/9b/Gustav_chocolate.jpg/168px-Gustav_chocolate.jpg" }); Pets.Add(new Animal { Name = "Arabian Mau", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/d/d3/Bex_Arabian_Mau.jpg" }); Pets.Add(new Animal { Name = "Bengal", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/thumb/b/ba/Paintedcats_Red_Star_standing.jpg/187px-Paintedcats_Red_Star_standing.jpg" }); Pets.Add(new Animal { Name = "Burmese", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/0/04/Blissandlucky11.jpg" }); Pets.Add(new Animal { Name = "Cyprus", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/thumb/b/b9/CyprusShorthair.jpg/320px-CyprusShorthair.jpg" }); Pets.Add(new Animal { Name = "German Rex", ImageUrl = "https://upload.wikimedia.org/wikipedia/commons/c/c7/German_rex_harry_%28cropped%29.jpg" }); } }
PetSearchHandler.cs public class PetSearchHandler : SearchHandler { protected override void OnQueryChanged(string oldValue, string newValue) { base.OnQueryChanged(oldValue, newValue); if (string.IsNullOrWhiteSpace(newValue)) { ItemsSource = null; } else { ItemsSource = PetData.Pets .Where(pet => pet.Name.ToLower().Contains(newValue.ToLower())) .ToList<Animal>(); } } protected override async void OnItemSelected(object item) { base.OnItemSelected(item); var pet = item as Animal; if (pet is null) return; await App.Current.MainPage.DisplayAlert(" ",pet.Name,"ok"); } }
添加一个Pets页面,在其中设置我们的PetSearchHandler
<?xml version="1.0" encoding="utf-8" ?> <ContentPage xmlns="http://xamarin.com/schemas/2014/forms" ... x:Class="HelloShell.Pets"> <Shell.SearchHandler> <controls:PetSearchHandler Placeholder="Enter search term" ShowsResults="true" DisplayMemberName="Name" /> </Shell.SearchHandler> <ContentPage.Content>
结果,我们应该在导航栏中获得一个带有搜索字段的页面,当您输入搜索短语时,将显示一个简单的宠物名称列表。
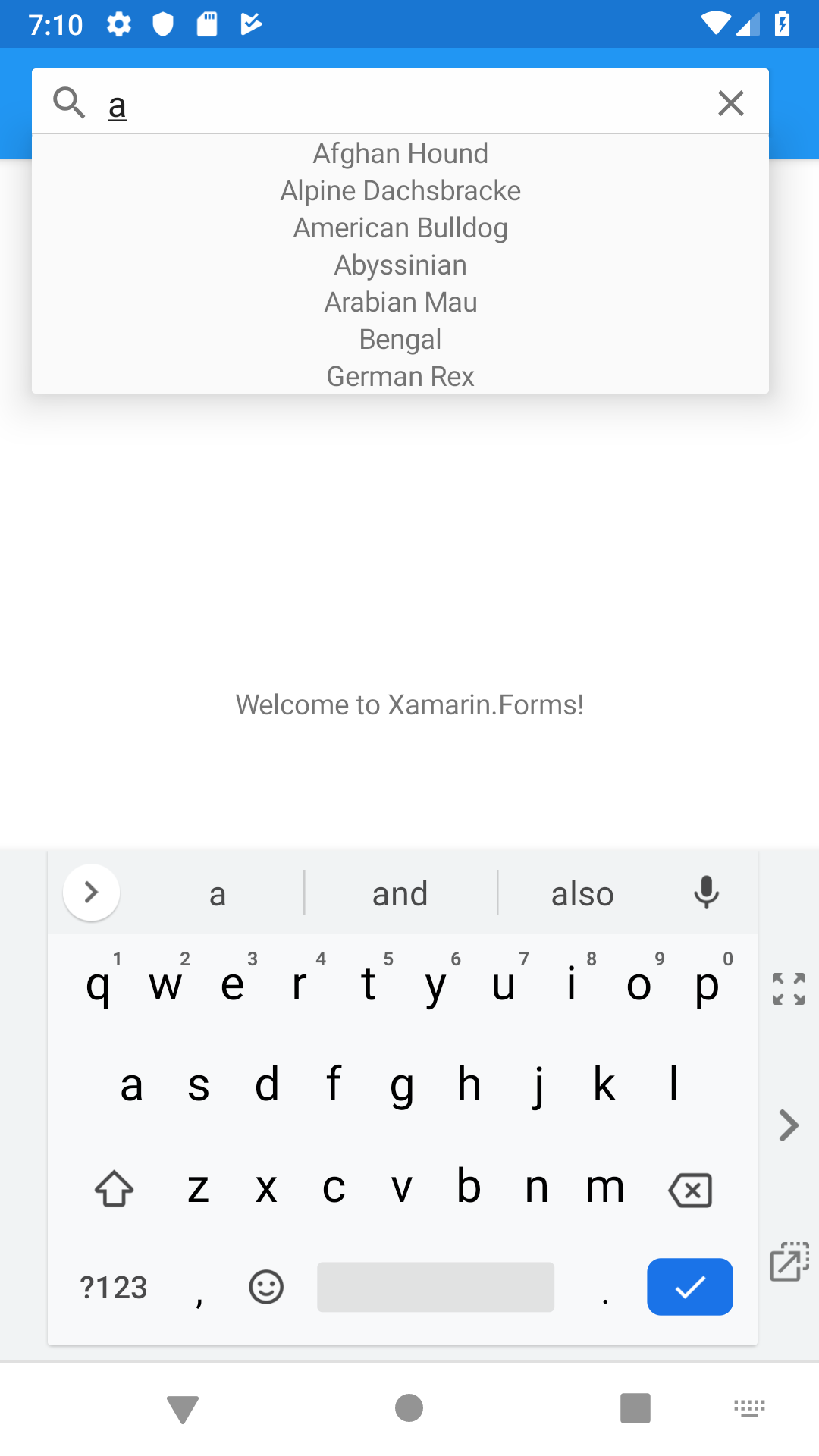
如果需要,我们可以通过在其中添加图片和几个文本标签来轻松自定义列表单元格的内容:
<Shell.SearchHandler> <controls:PetSearchHandler Placeholder="Enter search term" ShowsResults="true"> <controls:PetSearchHandler.ItemTemplate> <DataTemplate> <StackLayout Padding="10" Orientation="Horizontal"> <Image Source="{Binding ImageUrl}" Aspect="AspectFill" HeightRequest="40" WidthRequest="40" /> <Label Text="{Binding Name}" FontAttributes="Bold" /> </StackLayout> </DataTemplate> </controls:PetSearchHandler.ItemTemplate> </controls:PetSearchHandler> </Shell.SearchHandler>
github上的来源